Table of Contents
- Introduction to Three.js and Its Role in Web Development
- Setting Up Your Development Environment for Three.js
- Core Concepts in Three.js: Geometry, Materials, and Lighting
- Building Your First Simple Game with Three.js
- Advanced Techniques: Animation and Physics Integration
- Optimizing Performance for Browser-Based Games
- Publishing and Sharing Your Three.js Game with the World
Who this article is for:
- Web developers seeking to expand their skills in game development using Three.js
- Game designers and developers interested in creating 3D browser-based games
- Individuals exploring monetization and publishing opportunities for web-based games
Browser-based game development has undergone a quantum leap with Three.js, transforming what was once a domain of clunky Flash games into a powerhouse of immersive 3D experiences. The JavaScript library has demolished barriers between traditional desktop gaming and web applications, allowing developers to craft visually stunning games accessible from any device with a modern browser. Whether you’re a seasoned developer or taking your first steps into the world of interactive web applications, mastering Three.js unlocks a treasure trove of possibilities where your imagination becomes the only limitation. Ready to push the boundaries of what’s possible in the browser? Let’s dive into the world of Three.js game development.
Play free games on Playgama.com
Introduction to Three.js and Its Role in Web Development
Three.js stands as a high-level JavaScript library that abstracts WebGL’s complexity while providing powerful tools for creating 3D content in browsers. Founded by Ricardo Cabello (Mr.doob) in 2010, this open-source project has evolved into the cornerstone of browser-based 3D graphics.
At its core, Three.js serves as a bridge between raw WebGL capabilities and developer-friendly APIs. While WebGL offers direct access to the GPU for rendering graphics, its steep learning curve and verbose syntax make rapid development challenging. Three.js elegantly solves this problem by providing intuitive abstractions that maintain performance without sacrificing creative control.
If you’re looking to monetize your Three.js games after development, Playgama Partners offers an exceptional opportunity with up to 50% earnings from ads and in-game purchases. Their platform provides useful widgets, a complete game catalog, and flexible integration options through affiliate links. Learn more at https://playgama.com/partners.
The significance of Three.js in web development extends beyond mere graphical capabilities. It represents a paradigm shift in what browsers can deliver. Consider these core advantages:
- Cross-platform compatibility – Three.js games run on any device with a modern browser, eliminating the need for platform-specific development
- Zero-installation barrier – Users access your game instantly through a URL, removing friction typically associated with downloaded applications
- Direct distribution control – Bypass app stores and their revenue cuts by hosting games on your own infrastructure
- Continuous deployment – Update your game in real-time without requiring users to download patches
The ecosystem surrounding Three.js continues to grow, with community contributions extending its capabilities through plugins and extensions. From physics engines to advanced post-processing effects, these additions allow developers to incorporate sophisticated game mechanics without building everything from scratch.
Aspect | Three.js Approach | Benefits for Game Development |
Learning Curve | Moderate, based on JavaScript | Accessible to web developers without 3D expertise |
Performance | Optimized WebGL wrapper | Handles complex scenes with thousands of objects |
Community | Active, with 85k+ GitHub stars | Abundant resources, examples, and third-party extensions |
Integration | Works with major JS frameworks | Seamlessly incorporates into React, Vue, Angular projects |
Asset Support | Extensive format compatibility | Imports industry-standard 3D assets (glTF, OBJ, FBX) |
As WebGL continues to evolve and WebGPU emerges as the next generation of web graphics technology, Three.js remains positioned at the forefront, with active development ensuring compatibility with these advancements. This forward-looking approach makes Three.js not just a tool for today but an investment in the future of web-based game development.
Setting Up Your Development Environment for Three.js
Establishing an efficient development environment forms the foundation of any successful Three.js project. Unlike complex game engines requiring specialized software, Three.js development leverages standard web development tools with a few specialized additions.
Begin by setting up the essential components of your development stack. You have several installation options for incorporating Three.js into your project:
- NPM (recommended):
npm install three
- CDN inclusion:
<script src="https://cdn.jsdelivr.net/npm/[email protected]/build/three.min.js"></script>
- Direct download: from the official GitHub repository
For professional development, the NPM approach offers significant advantages through module bundling, version control, and seamless updates. This method integrates perfectly with build systems like Webpack or Vite, which optimize your final distribution package.
Sarah Jenkins, Senior Game Developer at Indie Studios
When I transitioned from Unity to Three.js for our company’s browser games, I initially struggled with the different approach to project organization. The breakthrough came when I structured my Three.js projects using a component-based system similar to what I was used to in Unity. I created wrapper classes for scene objects that handled their own behavior and state, then composed complex game entities from these components.
This approach transformed our development workflow—bugs became isolated to specific components, our team could work on different game elements simultaneously without conflicts, and onboarding new developers became significantly easier. Now our Three.js projects are as organized and maintainable as any native game engine project, but with the massive advantage of instant browser deployment.
The ideal development environment for Three.js extends beyond just including the library. Consider these essential tools:
- Code Editor: Visual Studio Code with the Three.js extension for syntax highlighting and intellisense
- Local Server: Essential for loading textures and models due to CORS policies (use Live Server VS Code extension)
- Build System: Vite provides exceptionally fast development with hot module replacement
- Version Control: Git for tracking changes and collaborative development
- 3D Asset Creation: Blender for creating and exporting compatible models
- Debugging Tools: Chrome DevTools with the Spector.js extension for WebGL inspection
For a streamlined project setup, consider using a template repository. Here’s a minimal project structure to establish clear organization from the beginning:
three-js-game/
├── src/
│ ├── index.js # Entry point
│ ├── game/
│ │ ├── Game.js # Main game class
│ │ ├── entities/ # Game objects
│ │ ├── systems/ # Physics, input, etc.
│ │ └── utils/ # Helper functions
│ └── assets/
│ ├── models/ # 3D models
│ ├── textures/ # Surface images
│ └── sounds/ # Audio files
├── public/ # Static files
│ └── index.html # HTML entry point
├── package.json # Dependencies
└── vite.config.js # Build configuration
To ensure optimal development efficiency, implement these practices from the start:
- Create reusable components for common game elements
- Establish a clear separation between game logic and rendering
- Implement a proper game loop with time-based updates instead of frame-based
- Use asset loading managers to handle asynchronous resource loading
- Configure proper development and production builds with environment-specific optimizations
When developing cross-platform Three.js games, consider Playgama Bridge—a unified SDK for publishing HTML5 games across different platforms. This solution streamlines deployment and ensures consistent performance across devices. Check out their comprehensive documentation at https://wiki.playgama.com/playgama/sdk/getting-started.
Core Concepts in Three.js: Geometry, Materials, and Lighting
Understanding the fundamental building blocks of Three.js is crucial for creating visually compelling and performant games. Three core elements form the backbone of any Three.js scene: geometry, materials, and lighting.
Geometry defines the shape and structure of 3D objects in your scene. Three.js provides built-in primitive geometries while supporting complex custom meshes:
- Primitives: BoxGeometry, SphereGeometry, CylinderGeometry, and TorusGeometry offer quick implementation for basic shapes
- BufferGeometry: The optimized base class for storing vertex data, critical for custom shapes and performance
- Imported Models: Complex geometries created in external software and imported via loaders (GLTFLoader being the industry standard)
When working with geometries, keep performance implications in mind. Every vertex and face impacts rendering speed, particularly on mobile devices. Techniques like geometry instancing allow reusing the same geometry across multiple objects while significantly reducing memory consumption and draw calls.
Materials determine how surfaces appear when rendered, controlling properties like color, texture, reflectivity, and transparency. Three.js offers several material types tailored to different rendering needs:
Material Type | Properties | Use Cases | Performance Impact |
MeshBasicMaterial | Not affected by lights | UI elements, performance-critical objects | Very low |
MeshStandardMaterial | Physically-based rendering | Realistic objects, main game elements | High |
MeshPhongMaterial | Shiny surfaces, specular highlights | Metallic objects, medium-quality rendering | Medium |
MeshLambertMaterial | Matte surfaces, no highlights | Non-shiny objects, performance optimization | Low |
ShaderMaterial | Custom GLSL shaders | Special effects, unique visual styles | Variable (depends on shader) |
Materials can be enhanced with textures to add visual detail without increasing geometry complexity. The TextureLoader in Three.js handles various image formats, while proper texture management (sizing to powers of two, mipmapping, texture atlases) profoundly affects both visual quality and performance.
Lighting brings scenes to life by providing depth, contrast, and atmosphere. Three.js offers several light types, each simulating different real-world lighting scenarios:
- AmbientLight: Provides uniform illumination to all objects without directional shadows
- DirectionalLight: Simulates distant light sources like the sun, casting parallel rays with shadows
- PointLight: Emits light in all directions from a single point, like a light bulb
- SpotLight: Projects a cone of light in one direction, perfect for flashlights or focused illumination
- HemisphereLight: Creates gradient lighting from sky to ground, ideal for outdoor environments
Shadow rendering, while visually impactful, demands significant processing power. Implement these optimizations for effective shadow usage:
// Configure optimized shadows for a directional light
const directionalLight = new THREE.DirectionalLight(0xffffff, 1);
directionalLight.position.set(5, 10, 7.5);
directionalLight.castShadow = true;
// Optimize shadow map settings
directionalLight.shadow.mapSize.width = 1024;
directionalLight.shadow.mapSize.height = 1024;
directionalLight.shadow.camera.near = 0.5;
directionalLight.shadow.camera.far = 50;
directionalLight.shadow.camera.left = -10;
directionalLight.shadow.camera.right = 10;
directionalLight.shadow.camera.top = 10;
directionalLight.shadow.camera.bottom = -10;
directionalLight.shadow.bias = -0.001;
// Add helper to visualize shadow camera (development only)
const helper = new THREE.CameraHelper(directionalLight.shadow.camera);
scene.add(helper);
The interplay between geometries, materials, and lighting creates the visual foundation of your Three.js game. Mastering these concepts enables you to balance visual fidelity with performance requirements—a critical skill for browser-based game development where device capabilities vary widely.
Building Your First Simple Game with Three.js
Creating your first game with Three.js demystifies the theoretical concepts we’ve covered by applying them to a concrete project. Let’s build a straightforward yet engaging 3D obstacle course where players navigate a sphere through a dynamically generated maze.
Start with establishing the foundational scene structure—the skeleton upon which your game will develop:
import * as THREE from 'three';
import { OrbitControls } from 'three/examples/jsm/controls/OrbitControls.js';
// Scene setup
const scene = new THREE.Scene();
scene.background = new THREE.Color(0x87CEEB);
// Camera configuration
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
camera.position.set(0, 15, 15);
camera.lookAt(0, 0, 0);
// Renderer initialization
const renderer = new THREE.WebGLRenderer({ antialias: true });
renderer.setSize(window.innerWidth, window.innerHeight);
renderer.shadowMap.enabled = true;
document.body.appendChild(renderer.domElement);
// Controls for development
const controls = new OrbitControls(camera, renderer.domElement);
// Lighting
const ambientLight = new THREE.AmbientLight(0xffffff, 0.5);
scene.add(ambientLight);
const directionalLight = new THREE.DirectionalLight(0xffffff, 0.8);
directionalLight.position.set(10, 20, 10);
directionalLight.castShadow = true;
scene.add(directionalLight);
// Game floor
const floorGeometry = new THREE.PlaneGeometry(30, 30);
const floorMaterial = new THREE.MeshStandardMaterial({
color: 0x66AA66,
roughness: 0.8,
});
const floor = new THREE.Mesh(floorGeometry, floorMaterial);
floor.rotation.x = -Math.PI / 2;
floor.receiveShadow = true;
scene.add(floor);
Next, implement the player character and basic physics using a simple gravity simulation:
// Player character
const playerGeometry = new THREE.SphereGeometry(0.5, 32, 32);
const playerMaterial = new THREE.MeshStandardMaterial({
color: 0x0099FF,
metalness: 0.3,
roughness: 0.4,
});
const player = new THREE.Mesh(playerGeometry, playerMaterial);
player.position.y = 0.5;
player.castShadow = true;
scene.add(player);
// Player physics state
const playerState = {
position: new THREE.Vector3(0, 0.5, 0),
velocity: new THREE.Vector3(0, 0, 0),
onGround: true,
speed: 0.05,
jumpForce: 0.15,
};
// Input handling
const keys = {};
document.addEventListener('keydown', (e) => {
keys[e.key] = true;
});
document.addEventListener('keyup', (e) => {
keys[e.key] = false;
});
Now, generate obstacles to create the gameplay challenge:
// Obstacle generation
function createObstacles() {
const obstacles = [];
for (let i = 0; i < 15; i++) {
const size = Math.random() * 3 + 1;
const height = Math.random() * 3 + 1;
const geometry = new THREE.BoxGeometry(size, height, size);
const material = new THREE.MeshStandardMaterial({
color: 0xDD4444,
roughness: 0.7,
});
const obstacle = new THREE.Mesh(geometry, material);
// Position randomly, but not on player start position
let validPosition = false;
while (!validPosition) {
obstacle.position.x = Math.random() * 25 - 12.5;
obstacle.position.z = Math.random() * 25 - 12.5;
obstacle.position.y = height / 2;
// Check distance from player start position
const distFromStart = new THREE.Vector2(obstacle.position.x, obstacle.position.z).length();
if (distFromStart > 3) {
validPosition = true;
}
}
obstacle.castShadow = true;
obstacle.receiveShadow = true;
scene.add(obstacle);
obstacles.push({
mesh: obstacle,
size: size,
height: height,
});
}
return obstacles;
}
const obstacles = createObstacles();
Finish with the game loop implementing collision detection, movement, and win condition:
// Game state
const gameState = {
active: true,
goal: new THREE.Vector3(10, 0.5, 10),
goalRadius: 1,
};
// Create goal
const goalGeometry = new THREE.CylinderGeometry(gameState.goalRadius, gameState.goalRadius, 1, 32);
const goalMaterial = new THREE.MeshStandardMaterial({
color: 0x00FF00,
emissive: 0x00FF00,
emissiveIntensity: 0.3,
});
const goal = new THREE.Mesh(goalGeometry, goalMaterial);
goal.position.copy(gameState.goal);
scene.add(goal);
// Game update function
function updateGame() {
if (!gameState.active) return;
// Handle player input
const moveDirection = new THREE.Vector3(0, 0, 0);
if (keys['ArrowUp'] || keys['w']) moveDirection.z -= playerState.speed;
if (keys['ArrowDown'] || keys['s']) moveDirection.z += playerState.speed;
if (keys['ArrowLeft'] || keys['a']) moveDirection.x -= playerState.speed;
if (keys['ArrowRight'] || keys['d']) moveDirection.x += playerState.speed;
if ((keys[' '] || keys['Space']) && playerState.onGround) {
playerState.velocity.y = playerState.jumpForce;
playerState.onGround = false;
}
playerState.velocity.x = moveDirection.x;
playerState.velocity.z = moveDirection.z;
// Apply gravity
if (!playerState.onGround) {
playerState.velocity.y -= 0.005; // Gravity
}
// Update position
playerState.position.add(playerState.velocity);
// Floor collision
if (playerState.position.y < 0.5) {
playerState.position.y = 0.5;
playerState.velocity.y = 0;
playerState.onGround = true;
}
// Obstacle collision
for (const obstacle of obstacles) {
// Simple box collision
if (
playerState.position.x > obstacle.mesh.position.x - obstacle.size/2 - 0.5 &&
playerState.position.x < obstacle.mesh.position.x + obstacle.size/2 + 0.5 &&
playerState.position.z > obstacle.mesh.position.z - obstacle.size/2 - 0.5 &&
playerState.position.z < obstacle.mesh.position.z + obstacle.size/2 + 0.5 &&
playerState.position.y < obstacle.height + 0.5
) {
// Push player out
const direction = new THREE.Vector3()
.subVectors(playerState.position, obstacle.mesh.position)
.normalize();
playerState.position.add(direction.multiplyScalar(0.1));
// Dampen velocity
playerState.velocity.x *= 0.8;
playerState.velocity.z *= 0.8;
}
}
// Boundary check
playerState.position.x = Math.max(-15, Math.min(15, playerState.position.x));
playerState.position.z = Math.max(-15, Math.min(15, playerState.position.z));
// Update player mesh position
player.position.copy(playerState.position);
// Check win condition
const distanceToGoal = playerState.position.distanceTo(gameState.goal);
if (distanceToGoal < gameState.goalRadius) {
gameState.active = false;
displayWinMessage();
}
}
function displayWinMessage() {
const winElement = document.createElement('div');
winElement.style.position = 'absolute';
winElement.style.top = '50%';
winElement.style.left = '50%';
winElement.style.transform = 'translate(-50%, -50%)';
winElement.style.padding = '20px';
winElement.style.background = 'rgba(0, 0, 0, 0.8)';
winElement.style.color = '#FFF';
winElement.style.fontSize = '24px';
winElement.style.borderRadius = '10px';
winElement.innerText = 'You Win! Refresh to play again.';
document.body.appendChild(winElement);
}
// Animation loop
function animate() {
requestAnimationFrame(animate);
updateGame();
controls.update();
renderer.render(scene, camera);
}
// Handle window resize
window.addEventListener('resize', () => {
camera.aspect = window.innerWidth / window.innerHeight;
camera.updateProjectionMatrix();
renderer.setSize(window.innerWidth, window.innerHeight);
});
animate();
This basic game demonstrates essential Three.js concepts: scene setup, object creation, user input, physics simulation, and game logic. While simplified, it contains the fundamental structure common to more complex games.
To expand this foundation, consider these enhancements:
- Add sound effects using the Web Audio API
- Implement a timer and scoring system
- Create multiple levels of increasing difficulty
- Add particle effects for jumping and collisions
- Incorporate more complex physics using a library like Ammo.js or Cannon.js
Remember that game development is iterative—start with a working minimal implementation, then expand features while maintaining performance and playability.
Advanced Techniques: Animation and Physics Integration
Moving beyond basic game mechanics, sophisticated Three.js games require advanced animation techniques and realistic physics simulation. These elements transform static scenes into dynamic, interactive experiences that respond naturally to player input.
Three.js provides built-in animation capabilities through its Animation system. This framework enables keyframe animation, skeletal rigging, and blending between different animation states—essential for character movement and environmental effects.
Michael Rodriguez, Technical Director
When our studio decided to create an in-browser multiplayer racing game using Three.js, we hit a major roadblock with physics. Our initial approach used a simple custom physics implementation that couldn’t handle the complex vehicle dynamics we needed.
The breakthrough came when we integrated Cannon.js with Three.js, creating a dual-representation system. Each game entity existed both as a visual Three.js object and a physical Cannon.js body, with a synchronization system keeping them aligned. We implemented a fixed timestep physics loop running separately from our rendering loop to ensure consistent physics behavior across different devices.
The results were remarkable—our vehicles exhibited realistic suspension, drifting, and collision responses while maintaining a solid 60fps on most devices. The separation of physics and rendering concerns also made our codebase significantly more maintainable, allowing us to iterate on gameplay without risking visual regressions.
To implement character animation with a skinned mesh, follow this approach:
import { GLTFLoader } from 'three/examples/jsm/loaders/GLTFLoader.js';
// Animation setup
let mixer;
const animationActions = {};
let activeAction;
// Load character model with animations
const loader = new GLTFLoader();
loader.load('models/character.glb', (gltf) => {
const model = gltf.scene;
scene.add(model);
// Set up animation mixer
mixer = new THREE.AnimationMixer(model);
// Store all animations
gltf.animations.forEach((clip) => {
const action = mixer.clipAction(clip);
animationActions[clip.name] = action;
// Optional: Adjust animation properties
action.clampWhenFinished = true;
action.loop = THREE.LoopRepeat;
});
// Set default animation
activeAction = animationActions['Idle'];
activeAction.play();
});
// Animation state management
function setAnimation(name, duration = 0.2) {
if (activeAction === animationActions[name]) return;
const nextAction = animationActions[name];
// Crossfade between animations
nextAction.reset();
nextAction.setEffectiveTimeScale(1);
nextAction.setEffectiveWeight(1);
activeAction.crossFadeTo(nextAction, duration, true);
activeAction = nextAction;
activeAction.play();
}
// Update animations in game loop
function updateAnimations(deltaTime) {
if (mixer) mixer.update(deltaTime);
}
For physics integration, Three.js games typically leverage dedicated physics engines. Among the popular options:
Physics Engine | Strengths | Ideal For | Integration Complexity |
Ammo.js | Full-featured port of Bullet physics | Complex simulations, vehicles | High |
Cannon.js | Lightweight, JavaScript-native | Medium complexity games | Medium |
Rapier | Modern, high-performance | Performance-critical applications | Medium |
Oimo.js | Simple API, small file size | Basic physics needs | Low |
Here’s how to integrate Cannon.js with Three.js for realistic physics:
import * as CANNON from 'cannon-es';
// Physics world setup
const world = new CANNON.World({
gravity: new CANNON.Vec3(0, -9.82, 0)
});
// Create physical ground
const groundBody = new CANNON.Body({
type: CANNON.Body.STATIC,
shape: new CANNON.Plane(),
});
groundBody.quaternion.setFromEuler(-Math.PI / 2, 0, 0);
world.addBody(groundBody);
// Create physical player
const playerRadius = 0.5;
const playerBody = new CANNON.Body({
mass: 5,
shape: new CANNON.Sphere(playerRadius),
position: new CANNON.Vec3(0, playerRadius, 0),
material: new CANNON.Material({ friction: 0.3, restitution: 0.3 }),
});
world.addBody(playerBody);
// Create physical obstacles
const obstaclesBodies = obstacles.map(obstacle => {
const halfExtents = new CANNON.Vec3(
obstacle.size / 2,
obstacle.height / 2,
obstacle.size / 2
);
const body = new CANNON.Body({
mass: 0, // Static body
shape: new CANNON.Box(halfExtents),
position: new CANNON.Vec3(
obstacle.mesh.position.x,
obstacle.mesh.position.y,
obstacle.mesh.position.z
),
});
world.addBody(body);
return body;
});
// Physics timestep variables
const fixedTimeStep = 1.0 / 60.0;
let lastCallTime;
// Update physics in game loop
function updatePhysics() {
const time = performance.now() / 1000;
if (!lastCallTime) {
world.step(fixedTimeStep);
} else {
const dt = time - lastCallTime;
world.step(fixedTimeStep, dt);
}
lastCallTime = time;
// Sync Three.js meshes with Cannon.js bodies
player.position.copy(playerBody.position);
player.quaternion.copy(playerBody.quaternion);
// Apply player movement forces
if (gameState.active) {
const moveForce = new CANNON.Vec3(0, 0, 0);
if (keys['ArrowUp'] || keys['w']) moveForce.z -= 40;
if (keys['ArrowDown'] || keys['s']) moveForce.z += 40;
if (keys['ArrowLeft'] || keys['a']) moveForce.x -= 40;
if (keys['ArrowRight'] || keys['d']) moveForce.x += 40;
playerBody.applyForce(moveForce);
// Jump if on ground
if ((keys[' '] || keys['Space']) && isPlayerOnGround()) {
playerBody.velocity.y = 7;
}
}
}
// Helper to detect if player is on ground
function isPlayerOnGround() {
let result = false;
// Cast ray downward from player position
const start = playerBody.position;
const end = new CANNON.Vec3(
start.x,
start.y - (playerRadius + 0.05), // Slightly below player
start.z
);
const ray = new CANNON.Ray(start, end);
ray.mode = CANNON.Ray.CLOSEST;
ray.checkCollisionResponse = true;
const result = ray.intersectWorld(world, {});
return result.hasHit;
}
Advanced animation techniques extend beyond character movement to environmental effects and UI elements:
- Particle systems for effects like fire, smoke, or magic using THREE.Points or dedicated libraries like three-nebula
- Procedural animation for elements like waving grass, flowing water, or dynamic clouds
- Vertex shader animations for efficient deformation effects without additional CPU overhead
- Post-processing effects using EffectComposer for bloom, motion blur, and other cinematic enhancements
To maintain performance while implementing these advanced features, structure your code to separate physics updates from rendering. A fixed timestep for physics ensures consistent behavior across different devices and frame rates, while animation updates can be tied to rendering for visual smoothness.
For developers looking to enhance their Three.js games with monetization features, Playgama Partners offers a seamless solution with earning potential of up to 50% on ads and in-game purchases. Their platform provides comprehensive tools for widget integration and distribution. Explore their partnership program at https://playgama.com/partners.
Optimizing Performance for Browser-Based Games
Performance optimization stands as the cornerstone of successful browser-based games. Unlike native applications, Three.js games must navigate the constraints of browser environments while delivering consistent experiences across a spectrum of devices—from high-end desktops to resource-constrained mobile phones.
Start with establishing performance metrics and measurement techniques. You cannot optimize what you cannot measure:
- Frames Per Second (FPS): Track using the Stats.js library for real-time monitoring
- Memory Usage: Monitor through Chrome DevTools’ Performance and Memory panels
- Draw Calls: Examine with Spector.js to identify rendering bottlenecks
- Asset Loading Time: Measure initial load and subsequent resource fetching
The renderer configuration provides your first opportunity for substantial performance gains:
// Adaptive renderer configuration
function configureRenderer() {
const isMobile = /iPhone|iPad|iPod|Android/i.test(navigator.userAgent);
const renderer = new THREE.WebGLRenderer({
antialias: !isMobile,
powerPreference: 'high-performance',
precision: isMobile ? 'mediump' : 'highp',
});
// Set pixel ratio with upper limit to prevent excessive rendering load
renderer.setPixelRatio(Math.min(window.devicePixelRatio, isMobile ? 2 : 3));
// Configure shadows based on device capability
renderer.shadowMap.enabled = true;
renderer.shadowMap.type = isMobile ?
THREE.BasicShadowMap :
THREE.PCFSoftShadowMap;
// Enable key performance features
renderer.physicallyCorrectLights = true;
renderer.outputEncoding = THREE.sRGBEncoding;
return renderer;
}
Geometry optimization fundamentally impacts performance through polygon count management. Implement these techniques:
- Level of Detail (LOD): Use THREE.LOD to swap lower-poly models at distance
- Geometry instancing: Apply THREE.InstancedMesh for repeated elements like trees, coins, or enemies
- Geometry merging: Combine static objects sharing the same material
- Occlusion culling: Skip rendering objects not visible to the camera
Material optimization complements geometry techniques:
// Performance-optimized material creation
function createOptimizedMaterial(config) {
const defaults = {
type: 'standard', // basic, standard, phong, lambert
color: 0xffffff,
textured: false,
texturePath: null,
textureSize: 1024,
glossiness: 0.5,
metalness: 0,
isMobile: /iPhone|iPad|iPod|Android/i.test(navigator.userAgent)
};
const options = { ...defaults, ...config };
let material;
// Select material type based on performance needs
switch(options.type) {
case 'basic':
material = new THREE.MeshBasicMaterial({ color: options.color });
break;
case 'lambert':
material = new THREE.MeshLambertMaterial({ color: options.color });
break;
case 'phong':
material = new THREE.MeshPhongMaterial({
color: options.color,
shininess: options.glossiness * 100
});
break;
case 'standard':
default:
material = new THREE.MeshStandardMaterial({
color: options.color,
roughness: 1 - options.glossiness,
metalness: options.metalness
});
break;
}
// Add texture if specified
if (options.textured && options.texturePath) {
const textureLoader = new THREE.TextureLoader();
const texture = textureLoader.load(options.texturePath);
// Apply texture optimization
texture.generateMipmaps = true;
texture.minFilter = THREE.LinearMipMapLinearFilter;
texture.magFilter = THREE.LinearFilter;
texture.anisotropy = options.isMobile ? 1 : 4;
material.map = texture;
}
// Further optimizations
material.flatShading = options.isMobile;
material.precision = options.isMobile ? 'mediump' : 'highp';
return material;
}
Scene management strategies improve both initial loading time and runtime performance:
- Asset streaming: Load assets progressively as needed rather than upfront
- Object pooling: Reuse objects instead of creating/destroying (particularly for projectiles, particles)
- Frustum culling: Utilize THREE.Frustum to determine visibility before rendering
- Scene partitioning: Divide large worlds into sectors loaded dynamically
Memory management prevents crashes and slowdowns, especially on mobile devices:
// Memory management utilities
const memoryManager = {
// Track disposable resources
resources: new Set(),
// Register resource for later disposal
track: function(resource) {
this.resources.add(resource);
return resource;
},
// Dispose specific resource
dispose: function(resource) {
if (!resource) return;
if (resource.dispose && typeof resource.dispose === 'function') {
resource.dispose();
}
if (resource.children) {
for (let i = resource.children.length - 1; i >= 0; i--) {
this.dispose(resource.children[i]);
}
}
if (resource.geometry) this.dispose(resource.geometry);
if (resource.material) this.dispose(resource.material);
if (resource.texture) this.dispose(resource.texture);
this.resources.delete(resource);
},
// Clear scene and dispose all resources
clearScene: function(scene) {
while(scene.children.length > 0) {
const object = scene.children[0];
scene.remove(object);
this.dispose(object);
}
},
// Texture-specific optimization
optimizeTexture: function(texture, size = 1024) {
if (!texture) return;
// Resize oversized textures
if (texture.image && (texture.image.width > size || texture.image.height > size)) {
const canvas = document.createElement('canvas');
const ctx = canvas.getContext('2d');
// Maintain aspect ratio
const ratio = texture.image.width / texture.image.height;
let newWidth, newHeight;
if (ratio > 1) {
newWidth = size;
newHeight = size / ratio;
} else {
newWidth = size * ratio;
newHeight = size;
}
canvas.width = newWidth;
canvas.height = newHeight;
ctx.drawImage(texture.image, 0, 0, newWidth, newHeight);
texture.image = canvas;
texture.needsUpdate = true;
}
// Optimize settings
texture.generateMipmaps = true;
texture.minFilter = THREE.LinearMipMapLinearFilter;
texture.anisotropy = renderer.capabilities.getMaxAnisotropy();
texture.needsUpdate = true;
return texture;
}
};
Adaptive quality settings based on device capability and performance metrics ensure consistent gameplay experience:
// Adaptive quality system
const qualityManager = {
currentFPS: 60,
targetFPS: 60,
qualityLevels: ['low', 'medium', 'high', 'ultra'],
currentQualityIndex: 2, // Start at 'high'
settings: {
low: {
shadowMapEnabled: false,
particleCount: 100,
drawDistance: 50,
textureSize: 512,
antialiasing: false
},
medium: {
shadowMapEnabled: true,
shadowMapType: THREE.BasicShadowMap,
particleCount: 300,
drawDistance: 100,
textureSize: 1024,
antialiasing: false
},
high: {
shadowMapEnabled: true,
shadowMapType: THREE.PCFShadowMap,
particleCount: 1000,
drawDistance: 200,
textureSize: 2048,
antialiasing: true
},
ultra: {
shadowMapEnabled: true,
shadowMapType: THREE.PCFSoftShadowMap,
particleCount: 3000,
drawDistance: 500,
textureSize: 4096,
antialiasing: true
}
},
// Initialize based on device detection
init: function() {
const isMobile = /iPhone|iPad|iPod|Android/i.test(navigator.userAgent);
const isHighEnd = (navigator.hardwareConcurrency || 0) >= 6;
if (isMobile) {
this.currentQualityIndex = isHighEnd ? 1 : 0; // medium or low
this.targetFPS = 30;
} else {
this.currentQualityIndex = isHighEnd ? 3 : 2; // ultra or high
}
this.applySettings();
},
// Apply current quality settings
applySettings: function() {
const quality = this.qualityLevels[this.currentQualityIndex];
const settings = this.settings[quality];
// Apply to renderer
renderer.shadowMap.enabled = settings.shadowMapEnabled;
if (settings.shadowMapEnabled) {
renderer.shadowMap.type = settings.shadowMapType;
}
// Anti-aliasing requires renderer recreation
if (renderer.antialias !== settings.antialiasing) {
// Store old properties
const oldSize = renderer.getSize(new THREE.Vector2());
const oldCanvas = renderer.domElement;
const parent = oldCanvas.parentElement;
// Create new renderer
renderer = new THREE.WebGLRenderer({ antialias: settings.antialiasing });
renderer.setSize(oldSize.x, oldSize.y);
// Replace the old canvas
if (parent) {
parent.removeChild(oldCanvas);
parent.appendChild(renderer.domElement);
}
}
// Update camera settings
camera.far = settings.drawDistance;
camera.updateProjectionMatrix();
// Update other game systems (example)
if (particleSystem) {
particleSystem.setMaxParticles(settings.particleCount);
}
// Log applied changes
console.log(`Applied ${quality} quality settings`);
},
// Monitor performance and adapt settings
update: function(deltaTime) {
// Update FPS measurement
this.currentFPS = 1 / deltaTime;
// Check for performance issues
if (this.currentFPS < this.targetFPS * 0.8 && this.currentQualityIndex > 0) {
// Reduce quality if FPS is below 80% of target
this.currentQualityIndex--;
this.applySettings();
}
else if (this.currentFPS > this.targetFPS * 1.2 &&
this.currentQualityIndex < this.qualityLevels.length - 1) {
// Increase quality if FPS is 20% above target
this.currentQualityIndex++;
this.applySettings();
}
}
};
These techniques collectively form a comprehensive performance optimization strategy for Three.js games. Implement them progressively, measuring impact at each stage to ensure your optimizations address the actual bottlenecks rather than theoretical concerns.
Publishing and Sharing Your Three.js Game with the World
Creating your Three.js masterpiece represents only half the journey—deploying it effectively ensures your game reaches its intended audience. The deployment process transforms your development project into a polished, accessible product ready for players worldwide.
Begin with preparing your project for production through the build process. Unlike development builds, production versions require optimization for size, loading speed, and compatibility:
// Example webpack configuration for production
module.exports = {
mode: 'production',
entry: './src/index.js',
output: {
filename: 'game.bundle.js',
path: path.resolve(__dirname, 'dist'),
},
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader',
options: {
presets: ['@babel/preset-env']
}
}
},
{
test: /\.(png|jpg|gltf|glb|mp3|wav)$/,
use: [
{
loader: 'file-loader',
options: {
name: '[name].[hash].[ext]',
outputPath: 'assets/',
},
},
],
},
],
},
optimization: {
minimizer: [
new TerserPlugin({
terserOptions: {
compress: {
drop_console: true,
},
},
}),
],
splitChunks: {
chunks: 'all',
},
},
plugins: [
new HtmlWebpackPlugin({
template: './public/index.html',
minify: {
collapseWhitespace: true,
removeComments: true,
removeRedundantAttributes: true,
},
}),
new CompressionPlugin({
test: /\.(js|css|html|svg|json)$/,
algorithm: 'gzip',
}),
],
};
The asset pipeline preparation ensures optimal delivery of 3D models, textures, and audio:
- Model optimization: Use tools like gltf-pipeline to compress and optimize 3D models
- Texture compression: Convert textures to efficient formats (WebP for diffuse maps, KTX2 with basis compression for others)
- Audio preparation: Compress audio to appropriate formats (.mp3 for music, .ogg for effects)
- Asset loading strategy: Implement progressive loading with THREE.LoadingManager
Hosting options vary based on project requirements and budget considerations:
Hosting Solution | Pros | Cons | Best For |
Static Hosts (Netlify, Vercel) | Free tier available, CI/CD integration, CDN distribution | Limited backend capabilities | Single-player games, portfolios |
GitHub Pages | Free, integrated with repositories | No backend support, limited bandwidth | Open-source projects, demos |
AWS/GCP/Azure | Scalable, full backend support, global reach | Higher complexity, potentially costly | Commercial games, multiplayer titles |
Traditional Web Hosting | Familiar workflow, often cheaper | Manual deployment, variable performance | Hobbyist projects, personal sites |
Game Portals (itch.io, Newgrounds) | Built-in audience, monetization options | Platform constraints, revenue sharing | Indie games seeking exposure |
Cross-browser compatibility remains crucial despite modern standards. Implement these measures to ensure broad accessibility:
// Browser compatibility check
function checkCompatibility() {
const issues = [];
// Check for WebGL support
if (!window.WebGLRenderingContext) {
issues.push('WebGL is not supported by your browser');
} else {
const canvas = document.createElement('canvas');
const gl = canvas.getContext('webgl') || canvas.getContext('experimental-webgl');
if (!gl) {
issues.push('WebGL is supported but disabled');
}
}
// Check for other required features
if (!window.localStorage) {
issues.push('localStorage is not supported (required for game saves)');
}
if (!window.AudioContext && !window.webkitAudioContext) {
issues.push('Web Audio API is not supported (game will run without sound)');
}
// Mobile-specific checks
const isMobile = /iPhone|iPad|iPod|Android/i.test(navigator.userAgent);
if (isMobile) {
// Check device memory if available
if (navigator.deviceMemory && navigator.deviceMemory < 2) {
issues.push('Device has limited memory. Performance may be affected.');
}
}
return {
compatible: issues.length === 0,
issues: issues
};
}
// Display compatibility UI if needed
const compatibilityResult = checkCompatibility();
if (!compatibilityResult.compatible) {
showCompatibilityWarning(compatibilityResult.issues);
}
function showCompatibilityWarning(issues) {
const warningEl = document.createElement('div');
warningEl.style.position = 'absolute';
warningEl.style.top = '0';
warningEl.style.left = '0';
warningEl.style.width = '100%';
warningEl.style.padding = '10px';
warningEl.style.backgroundColor = '#f8d7da';
warningEl.style.color = '#721c24';
warningEl.style.textAlign = 'center';
warningEl.style.zIndex = '1000';
let warningText = 'Compatibility issues detected: ';
warningText += issues.join(', ');
warningText += '. The game may not function correctly.';
warningEl.textContent = warningText;
document.body.appendChild(warningEl);
}
Monetization strategies offer various paths to generate revenue from your Three.js game:
- In-game advertising: Implement ad services compatible with HTML5 games
- Freemium model: Offer base gameplay free with premium features or content
- One-time purchase: Sell access through platforms like itch.io or your own payment system
- Subscription: Provide ongoing content updates for a recurring fee
- Sponsorship: Partner with brands for custom branded versions
When you're ready to deploy your Three.js game across multiple platforms, consider Playgama Bridge—a unified SDK designed specifically for HTML5 game cross-platform publishing. Their technology handles platform-specific adaptations automatically, saving you significant development time. Check out their comprehensive documentation at https://wiki.playgama.com/playgama/sdk/getting-started.
Analytics integration provides crucial insights into player behavior, enabling data-driven improvements:
// Game analytics implementation
const analytics = {
sessionId: null,
startTime: null,
events: [],
// Initialize analytics
init: function() {
this.sessionId = this.generateSessionId();
this.startTime = Date.now();
// Track session start
this.trackEvent('session_start', {
browser: navigator.userAgent,
screenSize: `${window.innerWidth}x${window.innerHeight}`,
isMobile: /iPhone|iPad|iPod|Android/i.test(navigator.userAgent),
language: navigator.language
});
// Setup automatic tracking
window.addEventListener('beforeunload', () => {
this.trackEvent('session_end', {
duration: (Date.now() - this.startTime) / 1000
});
this.sendBatch(true); // Force send on exit
});
// Periodic sending
setInterval(() => this.sendBatch(), 30000);
},
// Generate unique session ID
generateSessionId: function() {
return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function(c) {
const r = Math.random() * 16 | 0;
const v = c == 'x' ? r : (r & 0x3 | 0x8);
return v.toString(16);
});
},
// Track gameplay events
trackEvent: function(eventName, eventData = {}) {
const event = {
sessionId: this.sessionId,
timestamp: Date.now(),
event: eventName,
data: eventData
};
this.events.push(event);
},
// Send collected events to backend
sendBatch: function(immediate = false) {
if (this.events.length === 0) return;
// Clone events and clear queue
const eventsToSend = [...this.events];
this.events = [];
// Send data to analytics endpoint
fetch('https://your-analytics-api.com/collect', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
gameId: 'your-game-id',
events: eventsToSend
}),
// Only wait for response if immediate flag is set
keepalive: !immediate
}).catch(err => {
console.error('Analytics error:', err);
// Add events back to queue if sending failed
this.events = [...this.events, ...eventsToSend];
});
}
};
// Initialize analytics when game starts
analytics.init();
// Track game-specific events
function levelCompleted(levelId, score, timeSpent) {
analytics.trackEvent('level_completed', {
levelId,
score,
timeSpent
});
}
function playerDied(levelId, position, causeOfDeath) {
analytics.trackEvent('player_died', {
levelId,
position: {
x: Math.round(position.x * 100) / 100,
y: Math.round(position.y * 100) / 100,
z: Math.round(position.z * 100) / 100
},
causeOfDeath
});
}
function itemCollected(itemId, itemType) {
analytics.trackEvent('item_collected', {
itemId,
itemType
});
}
Marketing your Three.js game effectively requires strategic promotion across various channels:
- Game portals: Submit to WebGL showcases like itch.io, Newgrounds, and Kongregate
- Social media: Share development progress, gameplay videos, and GIFs
- Developer communities: Engage with Three.js and WebGL communities on Discord, Reddit, and forums
- Game jams: Participate in events like js13kGames or Ludum Dare for exposure
- Press outreach: Contact gaming and tech websites with press kits
Consider packaging your web game as a native application using technologies like Electron (desktop) or Capacitor (mobile). This approach provides additional distribution channels through app stores while maintaining your Three.js codebase.
Post-launch support extends the lifecycle of your game through:
- Regular content updates to maintain player interest
- Community engagement through Discord or forums
- Bug tracking and timely fixes based on user feedback
- Performance optimization for newly released devices
The publishing journey transforms your Three.js project from personal creation to public experience. A thoughtful approach to deployment, compatibility, analytics, and marketing maximizes your game's impact and potential for success.
The landscape of browser-based game development has fundamentally shifted with Three.js. The library bridges traditional boundaries between web applications and immersive gaming experiences, empowering developers to craft sophisticated 3D worlds accessible through a simple URL. By mastering the techniques covered—from core rendering concepts to advanced optimizations—you've gained the toolkit for creating games that rival native applications without sacrificing accessibility. The real power lies not just in the technology itself, but in how it democratizes game development, enabling your creative vision to reach players on any device with a modern browser. Your next masterpiece is only a render loop away.
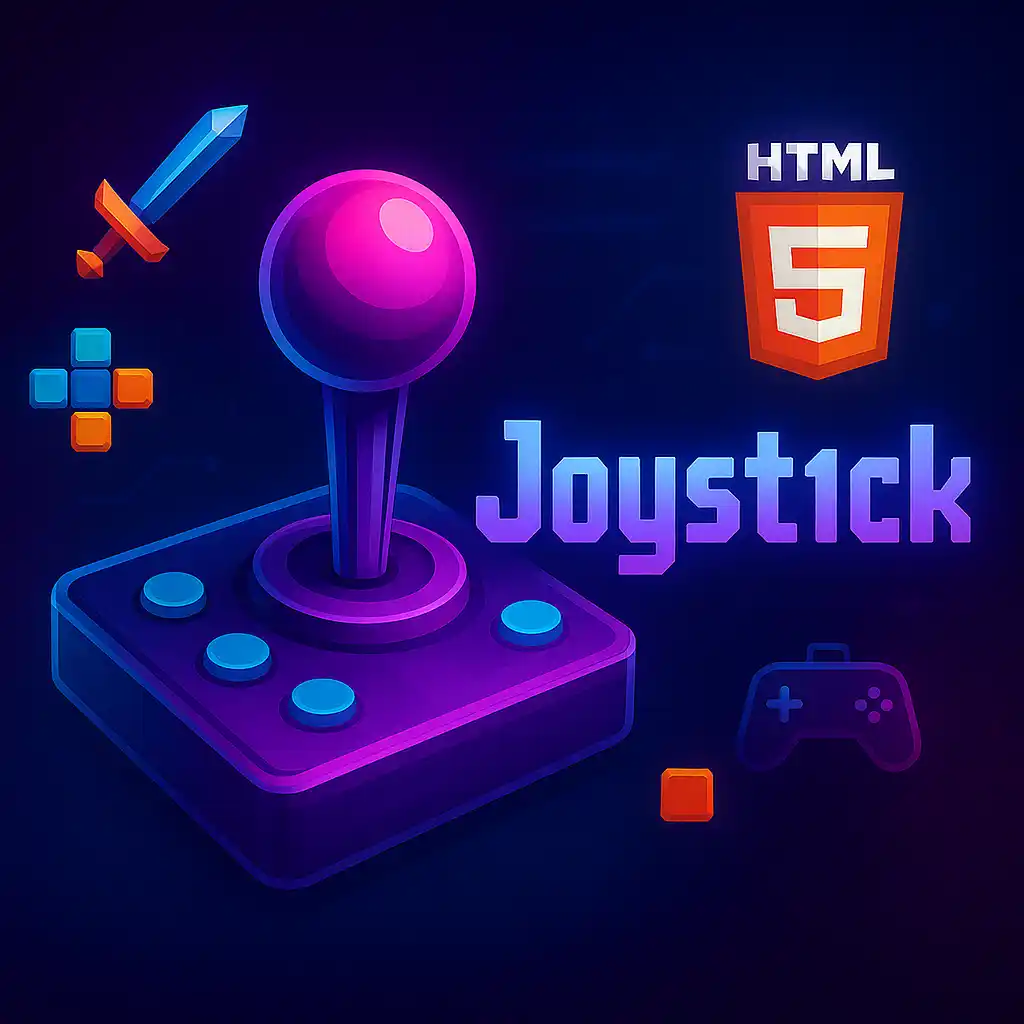