Table of Contents
Handling Mathematical Edge Cases in Game Simulation Engines
Managing mathematical edge cases, such as division by infinity, is crucial in simulation engines due to their potential impact on game logic and physics calculations. Here’s how you can address these challenges effectively:
1. Understanding Division by Infinity
In computational terms, division by infinity results in a value approaching zero but never actually reaching it. This is important when handling calculations that may result in such expressions, particularly in simulations and physics engines.
Play free games on Playgama.com
2. Implementing Safe Mathematical Operations
- Guard Clauses: Implement checks in your functions to handle potential infinity division cases. For example, use conditions to check if a divisor is extremely large or numerically infinite to return zero directly.
- Custom Error Handling: Design custom exceptions or error messages to catch and handle these cases gracefully without crashing the game engine.
function safeDivide(numerator, divisor) { if (divisor === Infinity) { return 0; } return numerator / divisor; }
3. Use Extended Real Number Libraries
Consider utilizing or developing libraries that implement the extended real number system, which can handle concepts like positive and negative infinity in a mathematically consistent manner.
4. Agent-Based Modeling Considerations
In agent-based simulations, ensure that agents deal with edge cases by incorporating strategies to adjust their behavior when encountering non-finite numbers.
5. Testing and Validation
- Unit Testing: Create specific test cases that verify the behavior of division operations against infinity and very large numbers.
- Simulation Consistency Checks: Regularly validate simulation outputs to ensure they adhere to expected mathematical behavior.
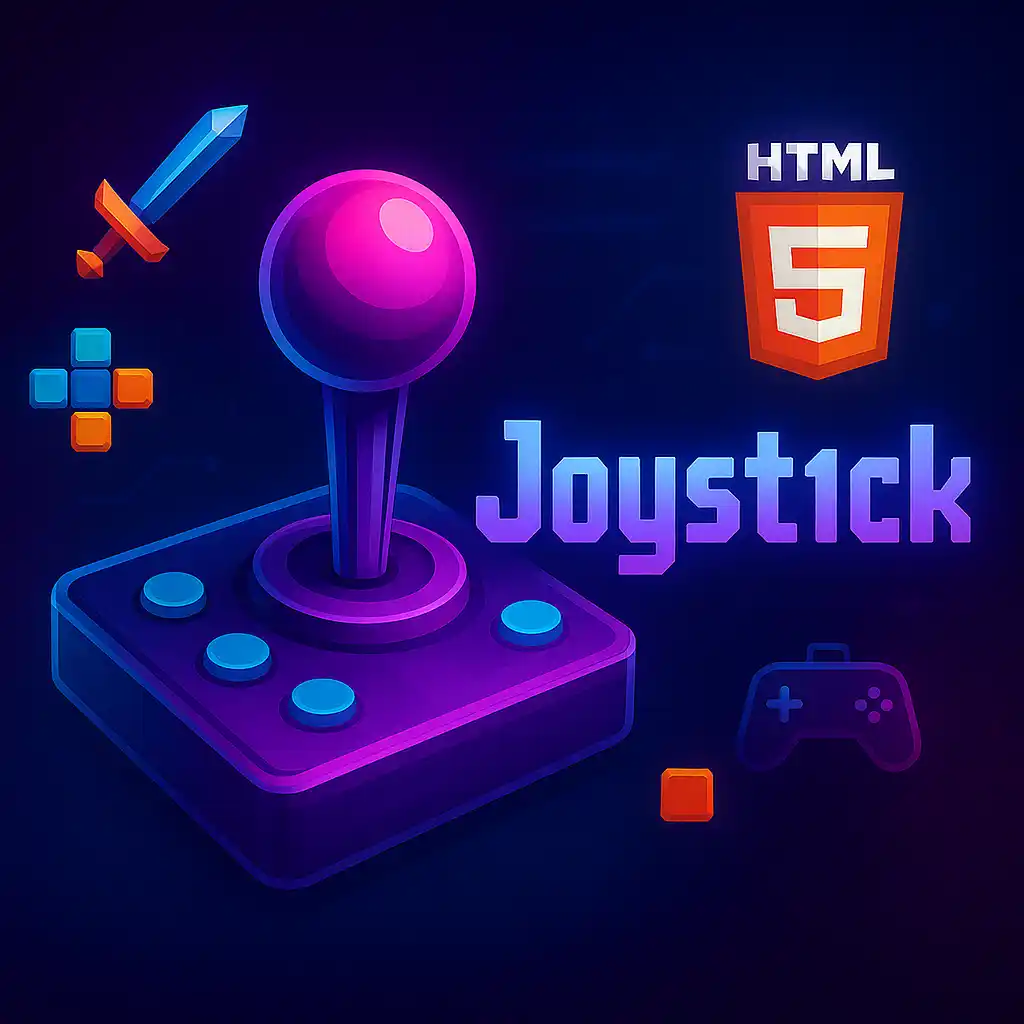