Table of Contents
Converting Degree Measurements to Radians in Game Engines
Rotations in game engines often involve calculations with angles, and it’s common to use radians instead of degrees. Radians are favored for mathematical precision and are the standard input for trigonometric functions like sin() and cos().
Why Use Radians?
- Mathematical Preference: Radians provide a direct proportion to arc length compared to degrees, making them more suitable for seamless mathematical operations.
- Native API Compatibility: Many game engine APIs and libraries expect input in radians, ensuring more consistent results across functions.
Conversion Formula
The formula to convert degrees to radians is:
Play free games on Playgama.com
radians = degrees * (Math.PI / 180)
Implementation in Different Languages
Here’s how you can implement this conversion in various programming languages used in game development:
Unity (C#)
float ConvertToRadians(float degrees) {
return degrees * Mathf.Deg2Rad;
}
Python
import math
def convert_to_radians(degrees):
return degrees * (math.pi / 180)
Godot (GDScript)
func convert_to_radians(degrees):
return deg_to_rad(degrees)
Ensuring Accuracy
Accuracy in conversions is paramount for precise rotations. Always use consistent data types and functions provided by the game engine for angle conversion to mitigate floating-point errors.
Practical Application in Games
For in-game rotations, such as character movement or camera angles, converting degrees to radians ensures compatibility with physics calculations and animation curves that inherently use radian inputs.
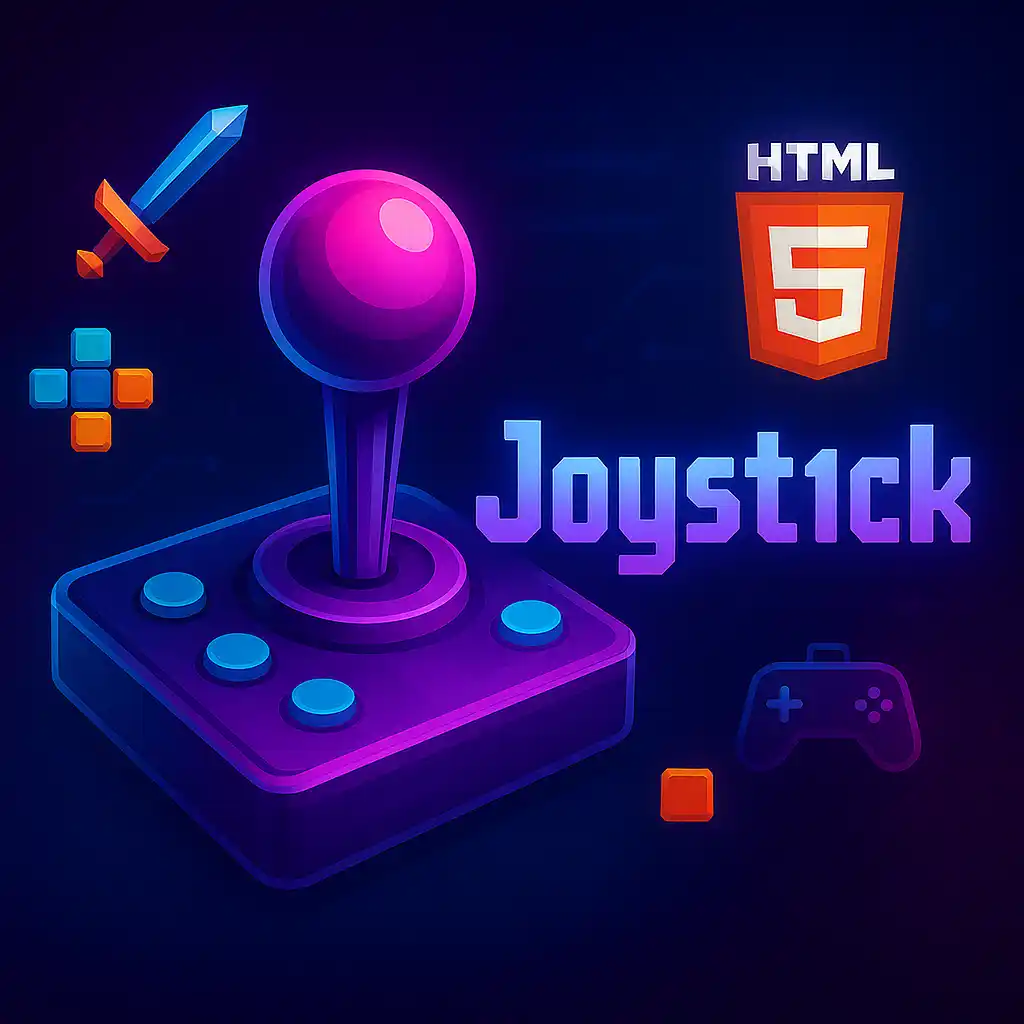