Table of Contents
Utilizing Lua for Scripting Game Mechanics
Why Use Lua?
Lua is a lightweight, high-level programming language designed primarily for embedded use in applications. Its simplicity and flexibility make it ideal for game development, especially in scripting game mechanics. Lua’s ease of embedding and great performance are perfect for real-time applications like games.
Embedding Lua into Your Game
- Integrate Lua with your game’s engine by utilizing Lua binding libraries like
lua-bind
orSol2
(for C++), which facilitate communication between the Lua interpreter and your game engine. - Enable your game engine to load and execute Lua scripts. This often involves setting up script managers that handle loading, running, and registering functions to Lua scripts.
// Example of loading a Lua script in C++
lua_State *L = luaL_newstate();
luaL_openlibs(L);
luaL_dofile(L, "path/to/your/script.lua");
Scripting Game Mechanics
- Create game-specific APIs in Lua that expose game mechanics. This will allow level designers and game devs to script mechanics such as player movement, enemy behavior, and more.
- Use Lua’s metatables to simulate complex data structures and manage game state effectively.
- Design scripts that respond to in-game events. Lua’s event-driven programming paradigm can handle callbacks for events like collisions, scores, and level completion.
-- Example Lua script for player movement
function movePlayer(x, y)
-- Assume player is a global or passed in entity
player:setPosition(x, y)
end
game:onUpdate(function(dt)
movePlayer(player:getX() + speed * dt, player:getY())
end)
Best Practices
- Performance Optimization: Avoid heavy computations in Lua scripts. Use Lua for high-level logic and leave the number-crunching to your game engine in C/C++.
- Script Organization: Organize scripts by roles or objects, such as player scripts, enemy scripts, GUI scripts, etc. This keeps the codebase modular and easier to maintain.
- Debugging Techniques: Utilize Lua’s debugging facilities, or third-party tools, to step through code, inspect values, and track script execution flow.
Real-World Examples
Lua has been successfully used in various games like World of Warcraft for UI customization and in Angry Birds to control gameplay elements. These examples show Lua’s versatility in handling game mechanics effectively.
Play free games on Playgama.com
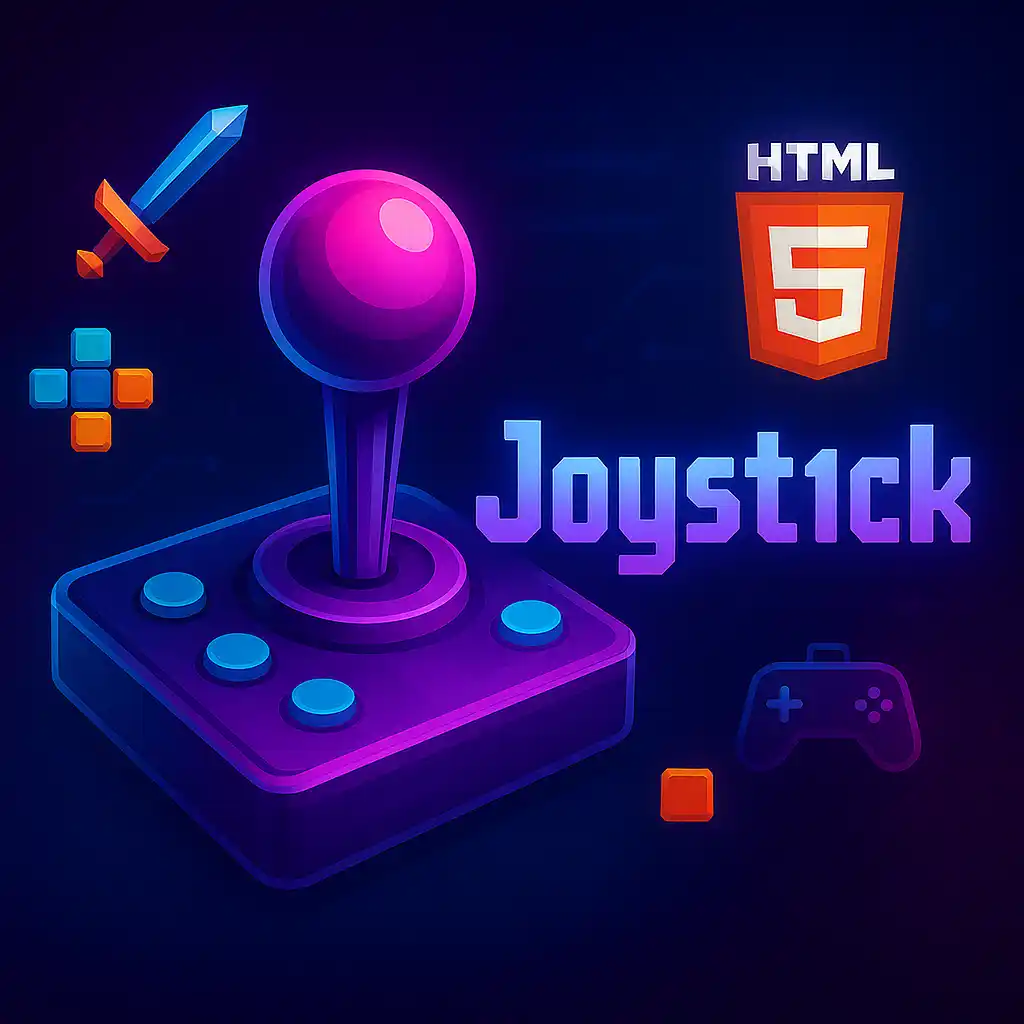