Table of Contents
Implementing Screen Rotation Functionality in iOS Games
To effectively support both landscape and portrait orientations in your iOS game, you need to handle screen rotation within the iOS environment using Objective-C and the iPhone SDK. Follow these steps to achieve a smooth transition between orientations:
1. Update Info.plist
Ensure that your app’s Info.plist file is configured to support the desired orientations. Add the UISupportedInterfaceOrientations
key with the necessary values such as UIInterfaceOrientationPortrait
and UIInterfaceOrientationLandscapeLeft
, among others, to declare supported orientations.
Play free games on Playgama.com
2. Implement Rotation Handling in UIViewController
- Override the
supportedInterfaceOrientations
method in your view controller to return the supported orientations:
- (UIInterfaceOrientationMask)supportedInterfaceOrientations { return UIInterfaceOrientationMaskAllButUpsideDown; }
- Use the
willRotateToInterfaceOrientation:duration:
method to prepare for orientation changes and adjust your view layout accordingly.
- (void)willRotateToInterfaceOrientation:(UIInterfaceOrientation)toInterfaceOrientation duration:(NSTimeInterval)duration { // Adjust game layout here }}
3. Respond to Orientation Changes
Implement viewWillTransitionToSize:withTransitionCoordinator:
to update your UI and game logic dynamically when the screen orientation changes:
- (void)viewWillTransitionToSize:(CGSize)size withTransitionCoordinator:(id<UIViewControllerTransitionCoordinator>)coordinator { [super viewWillTransitionToSize:size withTransitionCoordinator:coordinator]; [coordinator animateAlongsideTransition:^(id<UIViewControllerTransitionCoordinatorContext> context) { // Update game view on rotation } completion:nil]; }
4. Verify Automatic Orientation Handling
The iOS automatically handles the rotation; however, testing across different iOS devices ensures the game maintains visual quality and usability in both orientations.
By correctly implementing these steps, your mobile game will be equipped with robust multi-orientation support that enhances user experience on iOS devices.
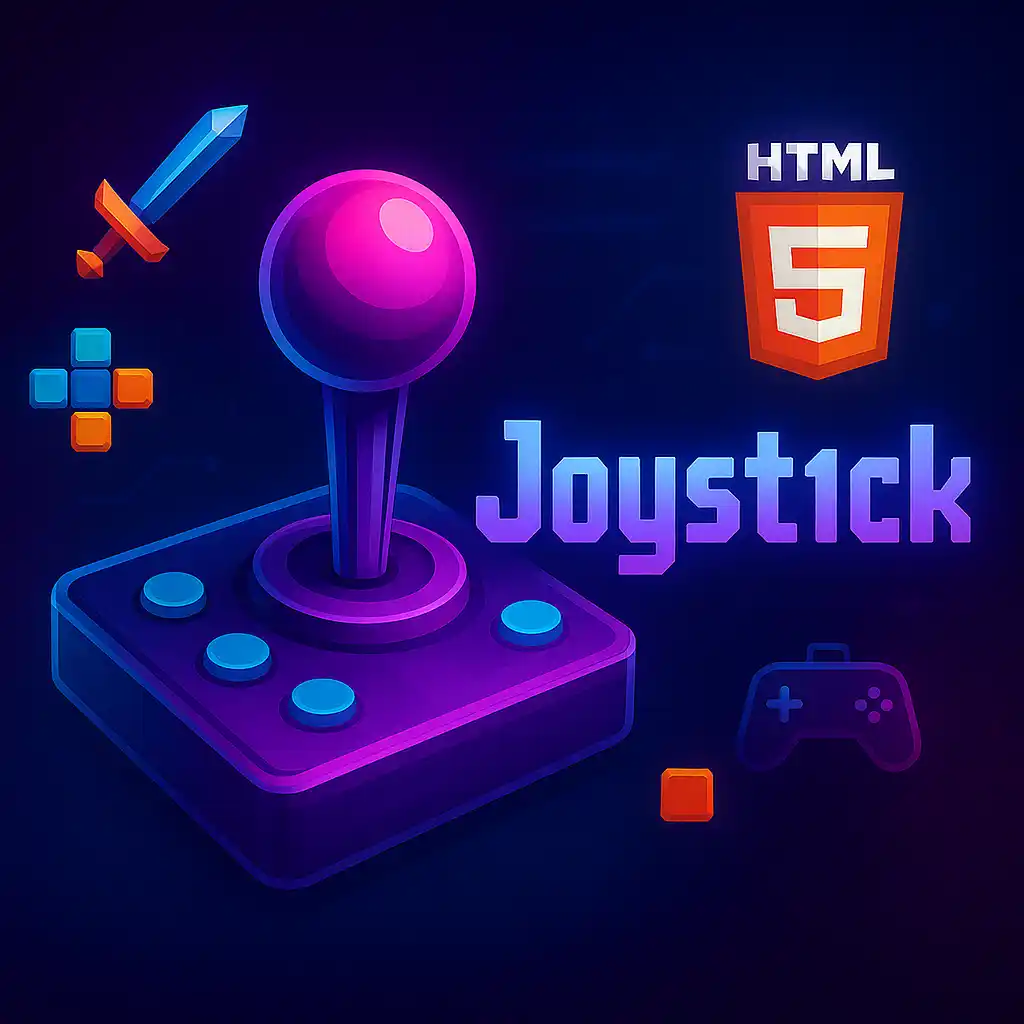