Table of Contents
Implementing Matrix Division in Graphics Engines
Matrix division, a concept often misunderstood, is not truly about dividing matrices as you would with scalars. Instead, it involves using matrix inversion and multiplication to achieve the desired computational result. In the context of a graphics engine, where transformations are paramount, understanding matrix division is crucial for carrying out efficient and correct transformations.
Matrix Inversion for Transformations
Matrix inversion is central to the concept of matrix division. Given a matrix, its inverse is the key to ‘dividing’ it by applying the inverse matrix in a multiplication operation:
Play free games on Playgama.com
if A * X = B, then X = A-1 * B
Here, A-1 is the inverse of matrix A. In graphics engines, matrix inversion is applied to transform matrices (like view or model matrices) to obtain an inverse transformation.
Efficient Matrix Operations for Graphics Engines
- Matrix Inversion: Not all matrices are invertible. A matrix must be square and have a non-zero determinant to have an inverse. Implementing inversion should check these conditions.
- Handling Non-Invertible Matrices: Introduce fallbacks or safe-fail mechanisms when dealing with non-invertible matrices, possibly reverting to other methods like pseudo-inversion.
- Optimization Techniques: Use row-reduction or adjoint method for smaller matrices common in transformations, such as 3×3 or 4×4 matrices.
Advanced Transformational Matrix Techniques
In advanced graphics programming, understanding how to efficiently implement matrices and their inverses can significantly enhance the rendering pipeline:
- Pre-computation: Pre-calculate common inverse matrices during the setup of your graphics engine to decrease run-time computations.
- Shader Programs: Offload matrix operations to GLSL or HLSL shader programs to leverage GPU parallelism.
- Matrix Caching: Cache previously computed inverse matrices in frames where the transformation doesn’t change, thus eliminating redundant computations.
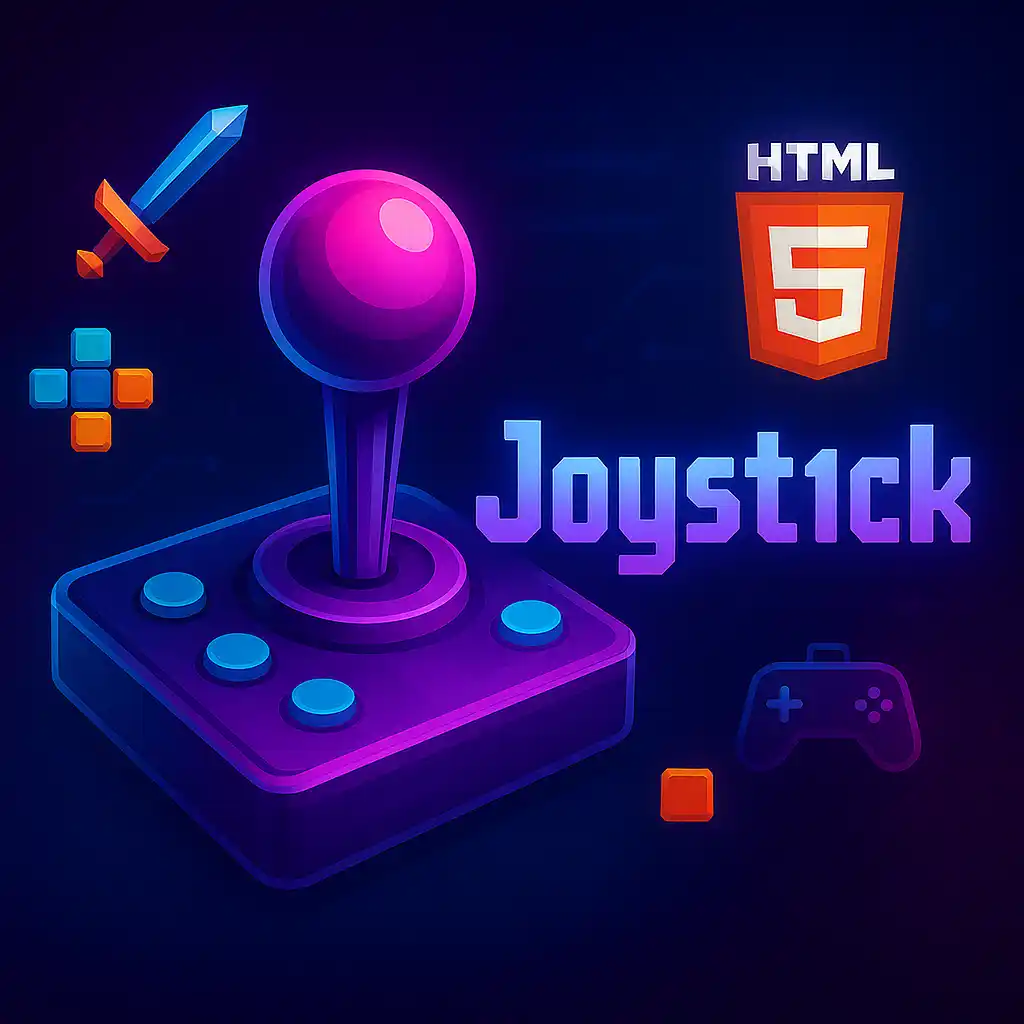