Table of Contents
Efficient Debugging Strategies in C++ using Console Output for Array Inspection
In C++ game development, efficient debugging is vital for performance and correctness. Inspecting arrays can be particularly challenging, but printing their contents to the console is a straightforward strategy. Here is a step-by-step guide to accomplish this efficiently:
1. Use of Console Output
Console output is a vital tool for debugging. It provides real-time feedback during game execution. The C++ standard library provides std::cout
for outputting data to the console.
Play free games on Playgama.com
2. Traversing Arrays
To print each element of an array, use a for
loop:
int array[] = {10, 20, 30, 40, 50}; int size = sizeof(array) / sizeof(array[0]); for(int i = 0; i < size; i++) { std::cout << "Element " << i << ": " << array[i] << std::endl; }
This method ensures all elements are displayed, helping identify issues such as incorrect values or out-of-bounds errors.
3. Enhanced Debugging with Modern C++ Features
Using modern C++ features like range-based for loops simplifies code:
for (const auto& element : array) { std::cout << element << std::endl; }
This method is not only more readable but also reduces the risk of index errors.
4. Conditional Debugging
To avoid excessive console output, especially in performance-sensitive areas, utilize conditional debugging techniques:
if(debugMode) { for(int i = 0; i < size; i++) { std::cout << "Element " << i << ": " << array[i] << std::endl; }}
By toggling debugMode
, you control when verbose output is activated, preserving performance during normal execution.
5. Tool-Assisted Debugging Strategies
Besides console debugging, leveraging IDE features like breakpoints and watch variables greatly improve debugging efficiency. Visual Studio, for instance, offers advanced debugging tools for C++ developers.
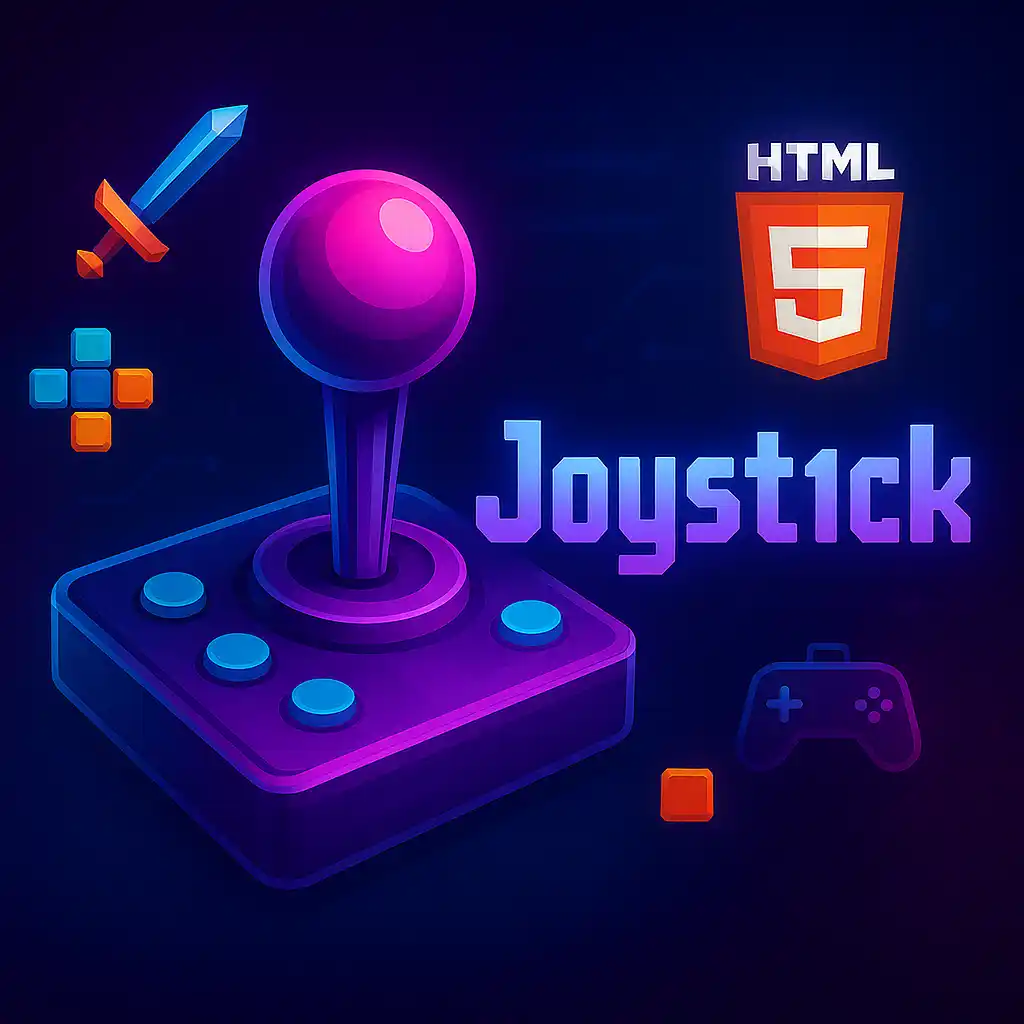