Table of Contents
- Understanding MelonJS and Its Capabilities
- Setting Up Your Development Environment
- Core Concepts of MelonJS Game Architecture
- Designing Engaging Game Mechanics with MelonJS
- Utilizing MelonJS for Visual and Audio Assets
- Tips for Optimizing Performance in MelonJS Games
- Publishing and Sharing Your MelonJS Game
Who this article is for:
- Independent game developers looking to create 2D games using HTML5.
- Web developers transitioning into game development who are familiar with JavaScript.
- Game designers and creators interested in utilizing the MelonJS engine for game production.
Game development has undergone a dramatic evolution, with independent developers now wielding incredible power to create enthralling experiences without massive studio resources. Standing at this intersection of accessibility and capability is MelonJS—a lightweight yet robust HTML5 game engine that empowers creators to bring their visions to life. Whether you’re crafting a nostalgic platformer or an innovative puzzle game, MelonJS offers the technical framework to transform your creative concepts into playable reality. This guide will navigate the essential elements of MelonJS development, from initial setup to final publication, equipping you with the knowledge to create genuinely captivating games that resonate with players across platforms.
Play free games on Playgama.com
Understanding MelonJS and Its Capabilities
MelonJS emerges as a standout option in the crowded marketplace of game engines due to its specialized focus on 2D game development within the HTML5 ecosystem. First released in 2011, this open-source engine has evolved into a mature platform, offering developers a comprehensive toolkit for creating browser-based games without the overhead of larger, more complex systems.
At its core, MelonJS leverages JavaScript—a language many web developers already know—making it particularly accessible for those transitioning from web development into game creation. The engine follows an entity-component pattern, providing a structured approach to game architecture while maintaining flexibility for custom implementations.
If you’re looking to monetize your MelonJS games effectively, Playgama Partners offers a partnership program with earnings of up to 50% on advertising and in-game purchases. You can add widgets, download a complete catalog of games, or add affiliate links to maximize your revenue streams. Learn more at https://playgama.com/partners.
The capabilities of MelonJS extend well beyond basic rendering. The engine provides advanced features that significantly reduce development time:
- Integrated physics engine with collision detection
- Sprite sheet support with animation management
- Tiled map editor integration for level design
- Comprehensive audio management
- Input handling across multiple platforms (keyboard, touch, gamepad)
- Particle system for visual effects
- State management for game screens and transitions
One of MelonJS’s standout attributes is its performance optimization for mobile platforms—crucial in today’s market where mobile gaming represents a significant portion of the industry. The engine intelligently manages resources to maintain smooth gameplay experiences even on less powerful devices.
Feature | MelonJS | Phaser | PixiJS |
Primary Focus | 2D Game Engine | 2D Game Engine | 2D Rendering Library |
Physics Engine | Built-in | Multiple options | Requires plugins |
File Size (minified) | ~520KB | ~800KB | ~270KB |
Learning Curve | Moderate | Low to Moderate | Moderate to High |
Tiled Integration | Native Support | Via plugins | Via plugins |
For developers seeking to create games that function across browsers with minimal adaptation, MelonJS presents a compelling option. Its architecture abstracts away many browser-specific implementations, allowing developers to focus on game logic rather than compatibility issues.
Michael Chen, Lead Game Developer at Indie Collective
When I first discovered MelonJS in 2020, I was skeptical about its capabilities compared to the more popularized engines. I had just left a AAA studio and wanted to create games independently without the corporate constraints. My first project was a simple platformer inspired by classic NES games, but with modern twists in mechanics.
What struck me was how quickly I could prototype concepts. Within days, I had a working character with physics, collectibles, and basic enemies. The Tiled integration proved invaluable—I could design levels visually and see them immediately in the game. This rapid iteration cycle allowed me to focus on what made the game fun rather than wrestling with technical implementations.
When we eventually released “Chrono Jumper” six months later, the game ran flawlessly across browsers and mobile devices. The performance optimizations in MelonJS meant we didn’t need separate codebases for different platforms. The game has since been played by over 300,000 people, and the codebase remains maintainable enough that we’ve added three major content updates.
Setting Up Your Development Environment
Establishing an efficient development environment forms the foundation of successful MelonJS game creation. Unlike more complex engines that require extensive setup procedures, MelonJS offers a streamlined process that gets developers coding quickly without sacrificing essential tools.
Begin by ensuring your system meets the minimal requirements: any modern browser with HTML5 support and a text editor or IDE of your choice. Visual Studio Code has emerged as a preferred option due to its excellent JavaScript support, extensions for syntax highlighting, and integrated terminal.
The core installation process follows these steps:
- Create a new project directory for your game
- Install Node.js and npm (if not already present)
- Initialize your project with
npm init
- Install MelonJS with
npm install melonjs --save
- Set up a basic project structure with HTML, CSS, and JavaScript files
For developers who prefer a more guided approach, MelonJS offers a boilerplate template that provides a pre-configured project structure. This template can be cloned directly from GitHub:
git clone https://github.com/melonjs/boilerplate.git my-game
cd my-game
npm install
npm start
This boilerplate includes Webpack configuration, enabling modern JavaScript features, hot reloading for efficient development, and optimized building for production deployment.
Beyond the core engine, several complementary tools enhance the MelonJS development experience:
- Tiled Map Editor: Essential for level design, enabling visual creation of game worlds
- TexturePacker: Optimizes sprite sheets for efficient resource management
- Audacity: For editing and optimizing audio files
- Chrome DevTools: Particularly the Performance and Memory tabs for debugging
- melonJS Debug Plugin: Provides real-time visualization of collision boxes, performance metrics, and entity inspection
Setting up version control from the project’s inception represents a critical best practice. Initialize Git within your project directory and commit regularly to maintain a history of your development process:
git init
git add .
git commit -m "Initial project setup with MelonJS"
For collaborative projects, consider establishing a structured branching strategy from the beginning, using feature branches for new gameplay elements and maintaining a stable main branch.
Development Tool | Purpose | Integration Method |
Webpack | Module bundling, development server | Included in boilerplate or manual config |
Babel | ES6+ transpiling | npm install @babel/core @babel/preset-env |
ESLint | Code quality and style enforcement | npm install eslint –save-dev |
melonJS Debug Panel | Real-time game debugging | Import from melonjs/plugins |
Tiled Map Editor | Level design | External application with .tmx export |
Core Concepts of MelonJS Game Architecture
MelonJS implements a structured architecture that balances flexibility with organization, enabling developers to create complex games while maintaining manageable codebases. Understanding these core architectural concepts proves essential before diving into implementation.
At the foundation of MelonJS lies the game loop—the heartbeat that drives all game functionality. This loop handles three critical phases: updating game state, drawing elements to the screen, and processing input. MelonJS abstracts these mechanisms behind a clean API, allowing developers to focus on game logic rather than low-level operations.
The engine adopts an entity-component pattern, though with its own implementation approach. Key architectural elements include:
- Game Objects: The base class for all visual elements in the game world
- Entities: Interactive game objects with properties like position, velocity, and collision
- Sprites: Visual representations with animation capabilities
- Containers: Groups that manage collections of related objects
- Screens: Game states like menus, levels, and cutscenes
- Renderable: Base drawing capability for visual objects
A typical MelonJS game initializes with a structure similar to this:
// Initialize the game engine
me.device.onReady(function() {
// Initialize video
if (!me.video.init(640, 480, {parent: "screen", scale: "auto"})) {
alert("Your browser does not support HTML5 canvas.");
return;
}
// Load resources
me.loader.preload(game.resources, function() {
// Define game screens
me.state.set(me.state.MENU, new game.TitleScreen());
me.state.set(me.state.PLAY, new game.PlayScreen());
// Start with the menu screen
me.state.change(me.state.MENU);
});
});
The entity system forms the cornerstone of most MelonJS games. Creating a custom entity involves extending the base Entity class and implementing specific behaviors:
game.PlayerEntity = me.Entity.extend({
init: function(x, y, settings) {
// Call the constructor
this._super(me.Entity, 'init', [x, y, settings]);
// Set physics properties
this.body.setVelocity(3, 15);
this.body.setFriction(0.4, 0);
// Set animation states
this.addAnimation("walk", [0, 1, 2, 3]);
this.addAnimation("jump", [4]);
this.setCurrentAnimation("walk");
},
update: function(dt) {
// Handle input
if (me.input.isKeyPressed('left')) {
this.body.vel.x -= this.body.accel.x * me.timer.tick;
this.flipX(true);
} else if (me.input.isKeyPressed('right')) {
this.body.vel.x += this.body.accel.x * me.timer.tick;
this.flipX(false);
} else {
this.body.vel.x = 0;
}
// Update entity animation and physics
this.body.update(dt);
me.collision.check(this);
// Return true if we moved or animated
return (this._super(me.Entity, 'update', [dt]) || this.body.vel.x !== 0 || this.body.vel.y !== 0);
},
onCollision: function(response, other) {
// Handle collisions
return true;
}
});
MelonJS’s state management system facilitates transitions between different game screens, from menus to levels to cutscenes. Each state encapsulates its own lifecycle methods, including initialization, updates, and cleanup:
game.PlayScreen = me.Stage.extend({
onResetEvent: function() {
// Load a level
me.levelDirector.loadLevel("level1");
// Add HUD
this.HUD = new game.HUD.Container();
me.game.world.addChild(this.HUD);
},
onDestroyEvent: function() {
// Remove HUD
me.game.world.removeChild(this.HUD);
}
});
For developers looking to expand their MelonJS games to multiple platforms efficiently, Playgama Bridge provides a unified SDK for publishing HTML5 games across various platforms. This streamlines your deployment process and ensures consistent performance across environments. Check out the comprehensive documentation at https://wiki.playgama.com/playgama/sdk/getting-started.
The collision system in MelonJS deserves special attention, as it handles both detection and response with configurable parameters. By implementing the onCollision method in entities, developers can create sophisticated interactions between game elements based on collision types and properties.
Designing Engaging Game Mechanics with MelonJS
Game mechanics constitute the interactive foundation of player engagement. With MelonJS, implementing these mechanics becomes a structured process that balances technical implementation with creative design. The most captivating games emerge when mechanics align perfectly with thematic elements, creating cohesive experiences that feel natural to players.
Beginning with player movement—the most fundamental mechanic in most games—MelonJS provides a physics system that handles acceleration, velocity, and friction. This system can be fine-tuned to achieve various movement styles:
// For a floaty character (like in a space game)
this.body.setVelocity(3, 3);
this.body.setFriction(0.1, 0.1);
this.body.setMaxVelocity(5, 5);
// For a precise platformer character
this.body.setVelocity(4, 15);
this.body.setFriction(0.4, 0);
this.body.setMaxVelocity(8, 20);
Input handling represents another critical aspect of game mechanics. MelonJS offers a flexible input system that supports keyboard, mouse, touch, and gamepad interactions. Binding inputs to actions makes code more maintainable and allows for easy control reconfiguration:
// Define controls
me.input.bindKey(me.input.KEY.LEFT, "left");
me.input.bindKey(me.input.KEY.RIGHT, "right");
me.input.bindKey(me.input.KEY.SPACE, "jump", true); // true for "action on press"
// Using bound controls in entity update
if (me.input.isKeyPressed("left")) {
// Move left
} else if (me.input.isKeyPressed("right")) {
// Move right
}
if (me.input.isKeyPressed("jump") && !this.body.falling && !this.body.jumping) {
this.body.vel.y = -this.body.maxVel.y * me.timer.tick;
this.body.jumping = true;
}
Beyond movement, implementing game-specific mechanics requires thoughtful design. Consider these structured approaches for common game mechancs:
- Collectible Systems: Create a base collectible entity class with shared behavior, then extend for specific types (coins, power-ups, keys)
- Combat Mechanics: Implement attack hitboxes as temporary entities with collision properties
- Puzzle Elements: Use event-driven architecture to connect related objects (buttons to doors, switches to platforms)
- Progression Systems: Maintain player state across levels using the me.save module
- Enemy AI: Implement state machines for predictable yet engaging enemy behavior patterns
For more complex mechanics, consider implementing a component system on top of MelonJS’s entity framework. This approach allows for greater code reusability and cleaner organization:
// Base component class
game.Component = Object.extend({
init: function(entity) {
this.entity = entity;
},
update: function(dt) {
// Override in child components
}
});
// Example AI component
game.AIPatrol = game.Component.extend({
init: function(entity, rangeX) {
this._super(game.Component, 'init', [entity]);
this.rangeX = rangeX;
this.startX = entity.pos.x;
this.direction = 1;
},
update: function(dt) {
if (this.entity.pos.x >= this.startX + this.rangeX) {
this.direction = -1;
this.entity.flipX(true);
} else if (this.entity.pos.x <= this.startX) {
this.direction = 1;
this.entity.flipX(false);
}
this.entity.body.vel.x = this.direction * this.entity.body.accel.x * me.timer.tick;
}
});
// Using components in an entity
game.EnemyEntity = me.Entity.extend({
init: function(x, y, settings) {
// ... normal entity init
// Add components
this.components = [];
this.components.push(new game.AIPatrol(this, 200));
},
update: function(dt) {
// Update all components
this.components.forEach(function(component) {
component.update(dt);
});
// Normal entity update
this.body.update(dt);
return true;
}
});
Game feel—often called "juice"—elevates mechanics from functional to memorable. MelonJS provides several features to enhance feedback:
- Screen shake:
me.game.viewport.shake(intensity, duration, direction)
- Particle effects:
me.ParticleEmitter
for impacts, explosions, and environmental effects - Tween animations:
me.Tween
for smooth property transitions - Time manipulation:
me.timer.tick
can be adjusted for slow-motion or speed-up effects - Camera effects:
me.game.viewport.fadeIn/fadeOut
for dramatic transitions
Sarah Johnson, Game Design Director
My team had been struggling with a puzzle platformer that looked great but simply wasn't engaging players during playtests. The mechanics were technically sound—we had jumping, climbing, and object interaction—but something crucial was missing. Players would try the game and walk away without that spark of excitement we were aiming for.
After a particularly disappointing playtest session, we stepped back to analyze what was happening. The issue wasn't in our code quality or visual design—it was in the feedback loop. Our mechanics lacked "juice." Using MelonJS's built-in tools, we implemented a comprehensive feedback system: particle bursts when collecting items, screen shake for impacts, bounce tweens for interactive elements, and subtle camera movements to follow action.
The transformation was immediate in our next playtest. Players started smiling, laughing at satisfying interactions, and most importantly—continuing to play. Our retention metrics jumped by 47% without changing any fundamental mechanics. The lesson was clear: technically correct implementation is only half the equation. The other half is how these mechanics communicate with the player through feedback, creating a conversation between game and player that keeps them engaged.
Utilizing MelonJS for Visual and Audio Assets
Visual and audio elements transform abstract game mechanics into immersive experiences. MelonJS provides robust systems for asset management, allowing developers to create atmospherically rich games while maintaining performance optimization.
The asset loading system in MelonJS centralizes resource management through a manifest approach. This pattern ensures efficient loading and memory management:
// Resource manifest
game.resources = [
// Images
{ name: "player_spritesheet", type: "image", src: "data/img/player.png" },
{ name: "tileset", type: "image", src: "data/img/tileset.png" },
{ name: "background", type: "image", src: "data/img/background.png" },
// Sprite atlases
{ name: "texture_atlas", type: "json", src: "data/img/texture.json" },
// Fonts
{ name: "PressStart2P", type: "image", src: "data/fnt/PressStart2P.png" },
{ name: "PressStart2P", type: "binary", src: "data/fnt/PressStart2P.fnt" },
// Maps
{ name: "level1", type: "tmx", src: "data/map/level1.tmx" },
// Audio
{ name: "background_music", type: "audio", src: "data/audio/", channel: 1 },
{ name: "jump_sound", type: "audio", src: "data/audio/", channel: 4 }
];
// Loading with progress feedback
me.loader.preload(game.resources, function() {
me.state.change(me.state.PLAY);
});
For sprite animation, MelonJS provides a straightforward yet powerful animation system. Sprite sheets can be defined with frame-by-frame animations or using texture atlases generated by tools like TexturePacker or ShoeBox:
// Using a sprite sheet with uniform frames
this.renderable = new me.Sprite(0, 0, {
image: me.loader.getImage("player_spritesheet"),
framewidth: 32,
frameheight: 48
});
// Adding animations
this.renderable.addAnimation("idle", [0]);
this.renderable.addAnimation("walk", [0, 1, 2, 3, 4, 5], 100); // 100ms per frame
this.renderable.addAnimation("jump", [6]);
this.renderable.setCurrentAnimation("idle");
// Conditionally changing animations
if (this.body.vel.y !== 0) {
if (!this.renderable.isCurrentAnimation("jump")) {
this.renderable.setCurrentAnimation("jump");
}
} else if (this.body.vel.x !== 0) {
if (!this.renderable.isCurrentAnimation("walk")) {
this.renderable.setCurrentAnimation("walk");
}
} else if (!this.renderable.isCurrentAnimation("idle")) {
this.renderable.setCurrentAnimation("idle");
}
Background elements create depth and atmosphere in games. MelonJS supports parallax backgrounds for creating multi-layered scenes with different scrolling rates:
// Creating a parallax background
me.game.world.addChild(new me.ParallaxBackground({
image: me.loader.getImage("background"),
repeatY: false,
speed: {x: 0.5, y: 0}
}), 1);
The Tiled Map Editor integration represents one of MelonJS's most powerful features. This external tool allows designers to create complex levels visually, then import them directly into the game:
- Create tilesets and maps in Tiled
- Export as TMX format
- Load in MelonJS with
me.levelDirector.loadLevel("level1");
- Access layer properties with
me.game.world.getChildByName("layerName");
This workflow separates level design from programming, enabling artists and designers to iterate on levels independently of code development.
Audio management in MelonJS supports both sound effects and music tracks. The audio system handles format detection, channel management, and fallbacks for broader compatibility:
// Playing background music with fade-in
me.audio.playTrack("background_music", 0.7); // 0.7 volume
// Playing a sound effect
me.audio.play("jump_sound");
// Advanced sound control
me.audio.play("explosion_sound", false, function() {
// Callback after sound completes
me.game.viewport.shake(8, 500);
}, 0.8); // 0.8 volume
Asset Type | Recommended Format | Optimization Tips |
Sprite Sheets | PNG with transparency | Power-of-two dimensions, minimize empty space |
Background Images | JPEG (no transparency) or PNG | Consider splitting into layers for parallax |
Audio Music | MP3, OGG (for coverage) | 128-192kbps for web, consider looping points |
Sound Effects | MP3, WAV, OGG | Shorter sounds, careful normalization |
Tiled Maps | TMX (XML or JSON) | Reuse tilesets across levels, use object layers |
For handling text and UI elements, MelonJS provides bitmap font support and a UI component system:
// Adding a bitmap text score display
this.scoreText = new me.BitmapText(10, 10, {
font: "PressStart2P",
text: "Score: 0",
size: 1.0
});
me.game.world.addChild(this.scoreText, 10);
// Updating text
this.scoreText.text = "Score: " + game.data.score;
Particle systems create dynamic visual effects that enhance game feel. MelonJS includes a powerful particle system for creating effects like explosions, fire, smoke, and environmental elements:
// Creating a particle emitter for a coin collection effect
var particleEmitter = new me.ParticleEmitter(this.pos.x, this.pos.y, {
width: 10,
height: 10,
image: me.loader.getImage("particle"),
totalParticles: 15,
gravity: 0.2,
angle: 0,
angleVariation: 6.283185307179586,
speed: 3,
speedVariation: 1.5,
minLife: 800,
maxLife: 1500
});
// Configure colors with keyframes
particleEmitter.addColorStop(0, "#FFFF00");
particleEmitter.addColorStop(0.5, "#FFD700");
particleEmitter.addColorStop(1, "#FFA500");
// Start emitting
particleEmitter.streamParticles();
me.game.world.addChild(particleEmitter, 10);
Tips for Optimizing Performance in MelonJS Games
Performance optimization stands as a critical factor in delivering smooth gameplay experiences across devices. MelonJS provides several techniques and built-in features to ensure games maintain consistent frame rates even on less powerful hardware.
Asset loading and management represent the first opportunity for optimization. Strategic approaches include:
- Texture atlases: Combine multiple sprites into single image files to reduce HTTP requests and GPU texture switches
- Progressive loading: Split resources across levels to minimize initial loading time
- Asset compression: Use tools like TinyPNG for images and audio compression utilities
- Preload critical assets: Ensure essential resources load first to reduce perceived loading time
- Cache control: Implement proper HTTP headers for browser caching
Rendering optimization techniques specific to MelonJS include:
// Enable double buffering for smoother rendering
me.video.init(800, 600, {
doubleBuffering: true
});
// Container sorting - only sort when necessary
myContainer.autoSort = false; // Disable automatic sorting
myContainer.sort(); // Sort manually when needed
// Limit the number of simultaneous particles
particleEmitter.totalParticles = Math.min(
particleEmitter.totalParticles,
me.device.isMobile ? 15 : 30
);
// Use sprite visibility culling
me.game.world.autoDepth = false; // Disable automatic depth sorting
myEntity.alwaysUpdate = false; // Only update when on screen
Memory management requires particular attention in JavaScript games. Effective practices include:
- Object pooling: Reuse objects instead of creating new instances
- Texture management: Unload unused textures when changing levels
- Event cleanup: Properly remove event listeners when destroying entities
- Limit closures: Be mindful of potential memory leaks from closures
- Garbage collection: Structure code to minimize garbage collection pauses
Implementing object pooling for frequently created/destroyed objects like projectiles, particles, or enemies:
// Define a pool for bullet entities
game.bulletPool = new me.Pool();
game.bulletPool.init = function() {
this.grow(30); // Pre-allocate 30 bullets
return this;
};
// Factory method for the pool
game.bulletPool.newInstance = function() {
return new game.BulletEntity(0, 0, {});
};
// Initialize the pool early
game.bulletPool.init();
// Using the pool to create a bullet
fireBullet: function(x, y, direction) {
var bullet = game.bulletPool.pull();
bullet.pos.x = x;
bullet.pos.y = y;
bullet.direction = direction;
bullet.alive = true;
me.game.world.addChild(bullet, 5);
},
// When bullet is no longer needed
onCollision: function() {
me.game.world.removeChild(this);
game.bulletPool.push(this); // Return to pool
}
Physics calculations can be resource-intensive. MelonJS allows for several optimizations in this area:
- Limit collision checks to relevant entities with grouping
- Use simpler collision shapes when possible
- Implement spatial partitioning for large levels
- Disable physics for decorative elements
// Set collision filtering
this.body.collisionType = me.collision.types.PLAYER_OBJECT;
this.body.setCollisionMask(
me.collision.types.WORLD_SHAPE |
me.collision.types.ENEMY_OBJECT
);
// Simplified collision for performance
this.body.addShape(new me.Rect(0, 0, 32, 48));
// Disable physics entirely for decorative elements
this.body.collisionType = me.collision.types.NO_OBJECT;
Device-specific optimizations allow games to adapt to the capabilities of the player's hardware:
// Detect device capabilities and adjust settings
if (me.device.isMobile) {
// Reduce visual effects for mobile
game.settings.particleCount = 10;
game.settings.shadowsEnabled = false;
game.settings.postProcessing = false;
} else {
game.settings.particleCount = 30;
game.settings.shadowsEnabled = true;
game.settings.postProcessing = true;
}
// Apply settings throughout the game
function createExplosion(x, y) {
var emitter = new me.ParticleEmitter(x, y, {
totalParticles: game.settings.particleCount,
// other settings...
});
// ...
}
Performance monitoring and profiling are essential for identifying bottlenecks. MelonJS provides tools to help with this process:
// Enable the built-in performance monitor
import { DebugPanel } from 'melonjs/plugins/debug/debugPanel.js';
if (process.env.NODE_ENV === 'development') {
// Install the debug panel
const debugPanel = new DebugPanel();
// Create a debug panel
window.onload = function() {
me.device.onReady(function() {
// Initialize at the end of the game initialization
debugPanel.show();
});
};
}
When troubleshooting performance issues, follow this systematic approach:
- Identify the problem using performance metrics (CPU, GPU, memory)
- Isolate the issue by selectively enabling/disabling features
- Profile specific functions to find hotspots
- Implement targeted optimizations
- Measure the impact of changes
- Test across different devices and browsers
Publishing and Sharing Your MelonJS Game
After developing your MelonJS game, publishing and distribution represent the crucial final steps in reaching your audience. The HTML5 foundation of MelonJS offers versatile deployment options across multiple platforms and distribution channels.
For web deployment, prepare your game with these essential steps:
- Bundle optimization: Use Webpack or other bundling tools to minimize file size
- Asset compression: Enable GZIP on your server and optimize all assets
- Code minification: Remove comments and whitespace while obfuscating variable names
- Cache configuration: Set appropriate cache headers for static assets
- CDN implementation: Consider content delivery networks for global distribution
If you're using the MelonJS boilerplate, the production build process handles many of these optimizations automatically:
npm run build
This command generates optimized files in the dist/ directory, ready for deployment to web servers.
Web hosting options for HTML5 games include:
- Game portals: itch.io, Newgrounds, Kongregate, or Game Jolt
- Self-hosted: Personal websites or cloud hosting services
- GitHub Pages: Free hosting for open-source projects
- Specialized HTML5 game platforms: GameDistribution, CrazyGames, or Poki
When your MelonJS game is ready for wider distribution, consider utilizing Playgama Bridge—a unified SDK designed specifically for publishing HTML5 games across multiple platforms. This streamlines your deployment process and ensures your game performs consistently across different environments, allowing you to focus on creating great games rather than managing platform-specific requirements. Explore the comprehensive documentation at https://wiki.playgama.com/playgama/sdk/getting-started.
For mobile deployment, several options exist for packaging HTML5 games as native applications:
// Install Cordova globally
npm install -g cordova
// Create a Cordova project
cordova create my-game-app com.example.mygame MyGame
// Move to the project directory
cd my-game-app
// Add platforms
cordova platform add android
cordova platform add ios
// Copy your built MelonJS game to www folder
// (Replace this with your actual build path)
cp -R ../my-melonjs-game/dist/* www/
// Build the app
cordova build
Alternative packaging solutions include:
- Capacitor: Ionic's solution for building cross-platform apps
- Electron: For desktop applications on Windows, macOS, and Linux
- PWA conversion: Implementing Progressive Web App features
- Commercial services: CocoonJS or App.io for simplified packaging
To make your game discoverable and appealing to potential players, focus on these promotional elements:
- Compelling screenshots: Capture key gameplay moments and visual highlights
- Gameplay trailer: Create a 30-60 second video showcasing core mechanics
- Clear description: Explain gameplay, features, and unique selling points
- Proper tagging: Use relevant genres and keywords for search optimization
- Responsive design: Ensure your game works well across device sizes
Monetization options for MelonJS games include:
- Premium model: One-time purchase on platforms like itch.io or app stores
- In-game advertisements: Integration with ad networks like Adsense or GameMonetize
- Microtransactions: In-game purchases for virtual goods or features
- Sponsorship: Partnerships with brands or game portals
- Patreon or donation: Direct support from players who enjoy your work
For analytics and player feedback, implement tracking systems to understand how players interact with your game:
// Simple Google Analytics integration
// (Add the GA script to your HTML first)
function trackEvent(category, action, label) {
if (typeof ga !== 'undefined') {
ga('send', 'event', category, action, label);
}
}
// Track game events
trackEvent('Game', 'Level Complete', 'Level 1');
trackEvent('Game', 'Death', 'Enemy Collision');
Post-launch maintenance remains crucial for long-term success. Establish a sustainable update cycle that includes:
- Bug fixes based on player feedback
- Performance improvements for problematic devices
- New content additions to maintain player interest
- Seasonal or event-based updates
- Community engagement through social media or forums
Finally, consider open-sourcing components or tools you've developed during your game's creation. Contributing back to the MelonJS community strengthens the ecosystem and builds your reputation as a developer.
The journey of creating captivating games with MelonJS extends far beyond technical implementation. By mastering the engine's architecture, optimizing performance across devices, and thoughtfully designing game mechanics that resonate with players, you've acquired the essential skills for independent game development. Each project becomes an opportunity to refine your craft and push creative boundaries. As you publish and share your creations, remember that the most memorable games emerge from the perfect synthesis of technical excellence and artistic vision—a balance that MelonJS is uniquely positioned to help you achieve.
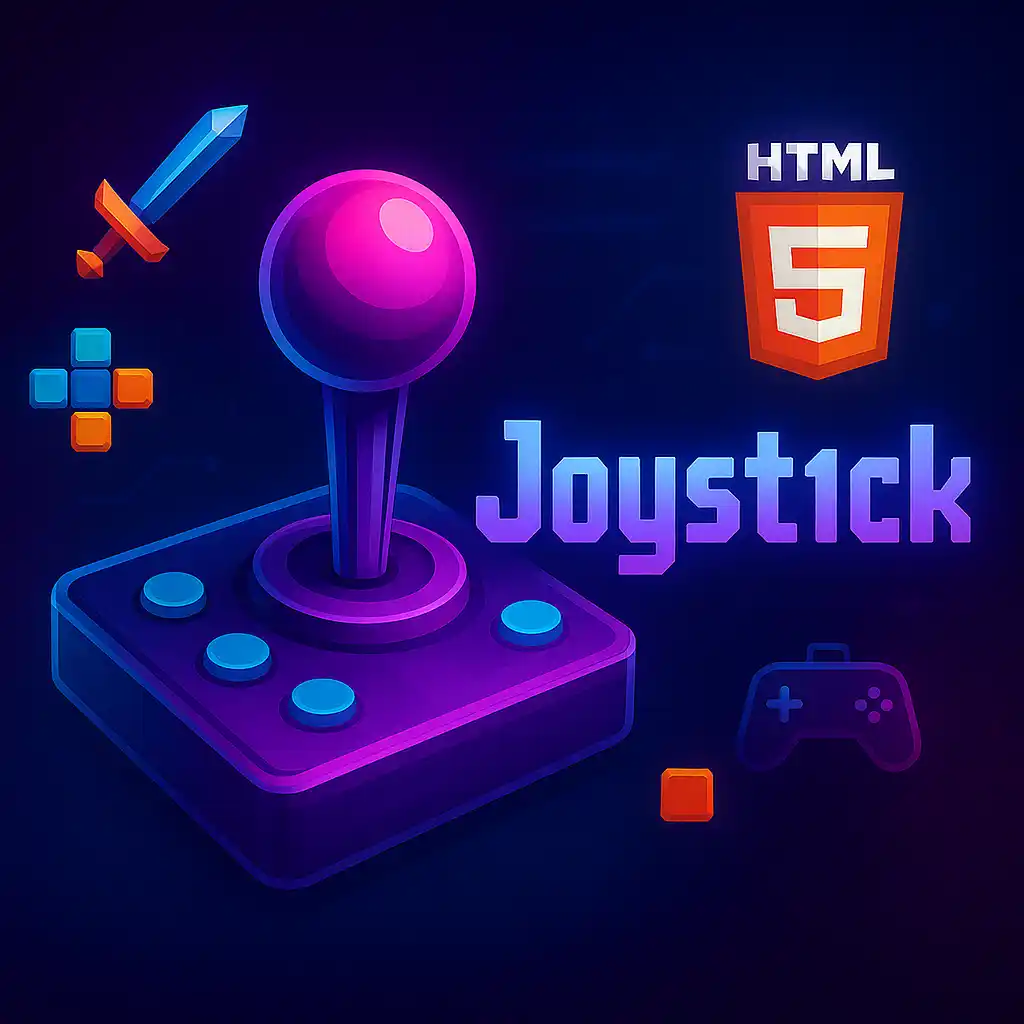