Table of Contents
Implementing AI for Peg Solitaire in Defold
Introduction to Peg Solitaire and Defold
Peg solitaire is a classic puzzle game where the goal is to clear the board of pegs by jumping one over another. Implementing AI for solving this puzzle in Defold, a versatile and light-weight game engine, involves a strategic approach leveraging AI algorithms.
Effective Algorithms for Peg Solitaire
- Depth-First Search (DFS): DFS explores all possible moves by delving deep into each path, making it efficient for exploring all potential game states in peg solitaire.
- Heuristic Search: By employing heuristics that prioritize moves leading towards the goal state—like maximizing pegs jumped—solvers can enhance efficiency.
- Dynamic Programming: Use DP to store previously computed results of board states, thereby avoiding redundant calculations and speeding up the solution process.
- Reinforcement Learning (RL): Algorithms like Deep Deterministic Policy Gradient (DDPG) can be trained to learn optimal strategies through trial and error, which is particularly useful in more complex configurations of peg solitaire.
Algorithm Implementation in Defold
In Defold, scripts are typically written in Lua, which easily supports the implementation of AI algorithms:
Play free games on Playgama.com
-- Example of a basic peg jump function in Lua
function peg_jump(board, source, target, over)
if board[over] == 1 and board[source] == 1 and board[target] == 0 then
board[target] = 1
board[over] = 0
board[source] = 0
return true
end
return false
end
AI Strategy Implementation Steps
- Set up a board representation in Defold using tables or arrays.
- Develop the movement logic using Lua to transition between board states.
- Incorporate a search algorithm (DFS/Heuristic) to sequence through potential moves.
- Integrate machine learning models like DDPG to enhance AI decision-making for complex patterns.
Testing and Optimization
Test the AI by simulating numerous game scenarios within Defold’s editor. Analyze performance metrics and adjust algorithms for optimal gameplay experience.
By adopting these strategies, developers can build efficient AI solutions for peg solitaire challenges using Defold.
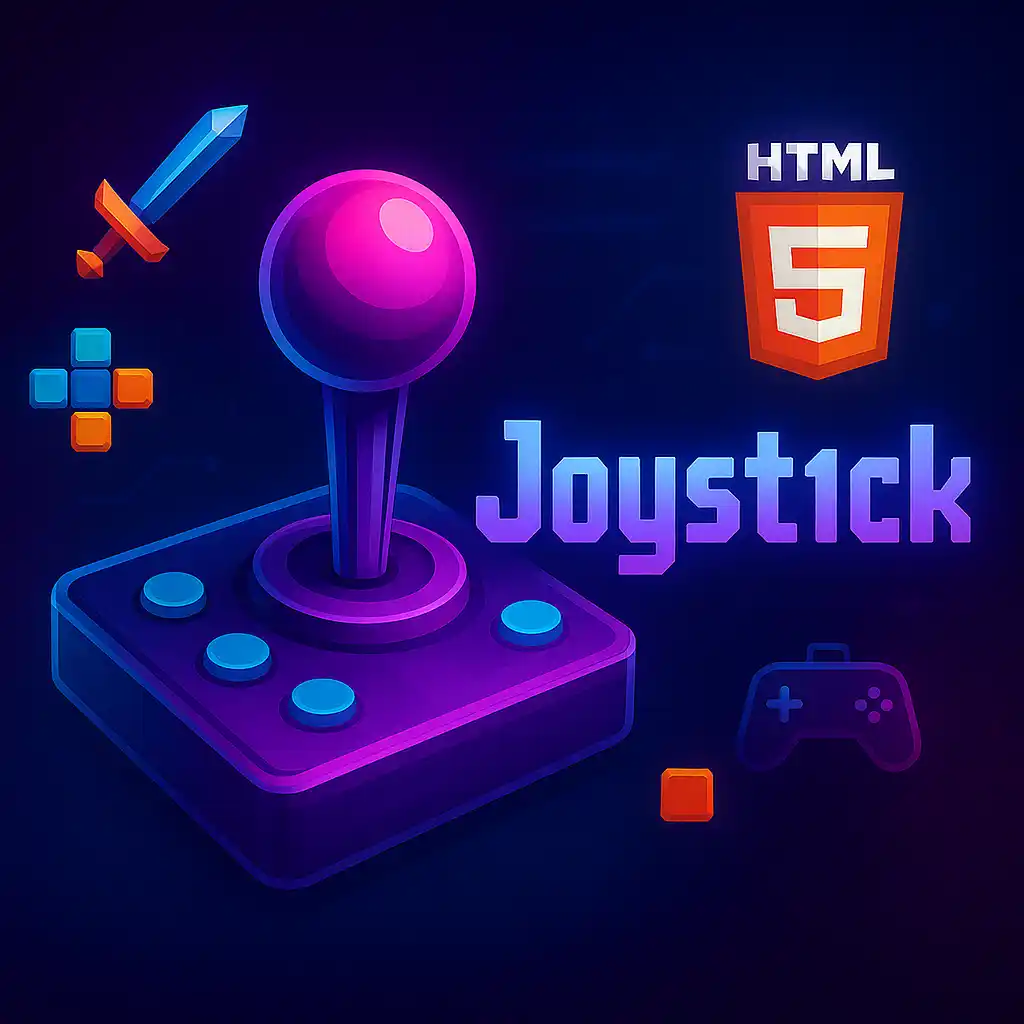