Table of Contents
Maintaining Object Rotation Data in 3D Game Engines
When developing with 3D game engines like Defold, ensuring the integrity of object rotation data is crucial for smooth gameplay and consistent object behavior. Here are steps and techniques to troubleshoot and prevent issues with rotation data:
1. Validate Data Persistence
Ensure that your game engine correctly saves and loads rotation data:
Play free games on Playgama.com
- Use persistent storage features of your engine for saving rotation states, like player preferences or game save files.
- Before runtime, test loading and saving sequences thoroughly to catch any anomalies early.
2. Utilize Quaternions
For rotation, use quaternions over Euler angles to prevent issues like gimbal lock:
- Quaternions represent rotations in 3D space effectively without the limitations of Euler angles.
- Most 3D engines, including Defold, provide built-in functions to handle quaternion operations.
- Example:
local q_rotation = vmath.quat_rotation_x(math.rad(angle))
3. Incorporate Error Handling
Implement error handling in your code to catch unexpected rotations:
- Use try/catch blocks (or language-specific equivalents) to manage rotational data read/write operations.
- Log any errors to a persistent log for post-mortem analysis.
4. Use Debugging Tools
Engage debugging tools within your engine to monitor real-time changes and data flow:
- Debug overlays or console outputs can provide immediate feedback on object states.
- Integrate breakpoints and watch variables related to object rotations during critical game flow segments.
5. Optimize for Performance
High-performance optimization can prevent loss of rotation data due to lag or processing hiccups:
- Profile the game’s performance and identify potential bottlenecks.
- Optimize assets and scripts to reduce computational overhead, ensuring the engine handles real-time data more efficiently.
6. Test Across Platforms
Rotation data issues might behave differently on various platforms:
- Conduct thorough testing on all target platforms to ensure consistent data integrity.
- Use platform-specific tools to assist in debugging and logging rotation data on each platform type.
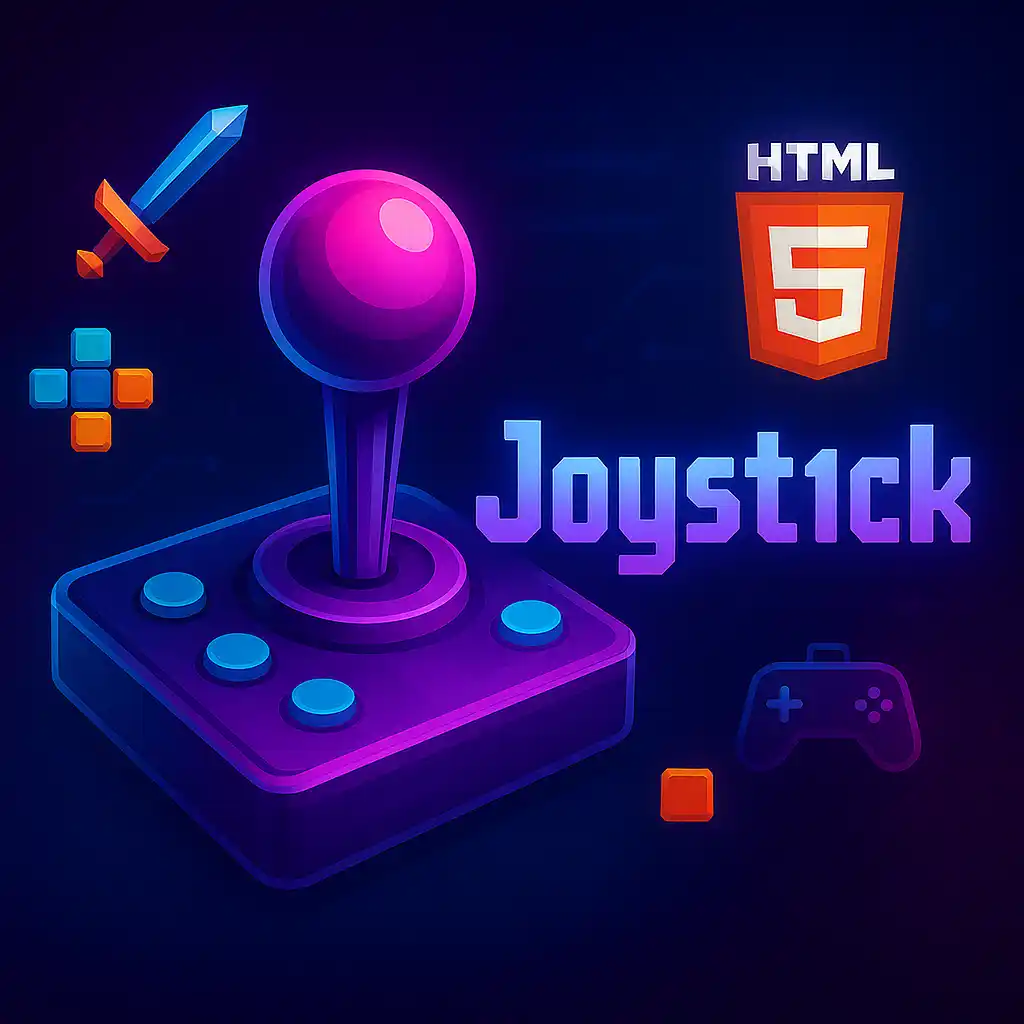