Table of Contents
Optimizing Foreground Sync Notifications in Android Mobile Games
Understanding Foreground Services
Foreground services are used in Android to perform tasks that are noticeable to the user, such as music playback or real-time data syncing. In gaming, they can be employed for scenarios like real-time notifications or data management, but careful implementation is crucial to avoid disrupting the gaming experience.
Key Strategies for Optimization
- Asynchronous Processing: Use asynchronous tasks to handle sync operations in the background, minimizing impact on the game’s main thread. Consider employing
AsyncTask
orWorkManager
for efficient background processing. - Optimized Use of Broadcast Receivers: Implement broadcast receivers for listening to system events and changes. Use them judiciously to update game state or notify the player without directly interfering with gameplay.
- Throttle Data Sync: To prevent excessive CPU usage and battery drain, throttle data sync operations by batching updates or using incremental sync processes.
- Efficient Network Usage: Use network libraries like Retrofit or OkHttp, which provide efficient network handling and background threading capabilities. They can help manage sync operations without affecting gameplay performance.
- Prioritize Game Thread: Ensure that all performance-intensive operations are offloaded from the main game thread. Use Unity’s
Job System
orTask.Run
in plain Android development to maintain smooth gameplay.
Example Code Snippet
public class SyncService : Service { @Override public int onStartCommand(Intent intent, int flags, int startId) { // Start a background thread for syncing new Thread(new Runnable() { public void run() { performSyncOperation(); } }).start(); return START_STICKY; } private void performSyncOperation() { // Perform sync task with minimal CPU impact } }
Testing and Monitoring
- Profile Performance: Use Android Studio’s profiler to monitor CPU, memory, network, and energy consumption during sync operations to identify bottlenecks.
- User Feedback and Metrics: Incorporate analytics to track user feedback and behavior during sync operations, ensuring that gamers are not experiencing noticeable lags or frame drops.
Play free games on Playgama.com
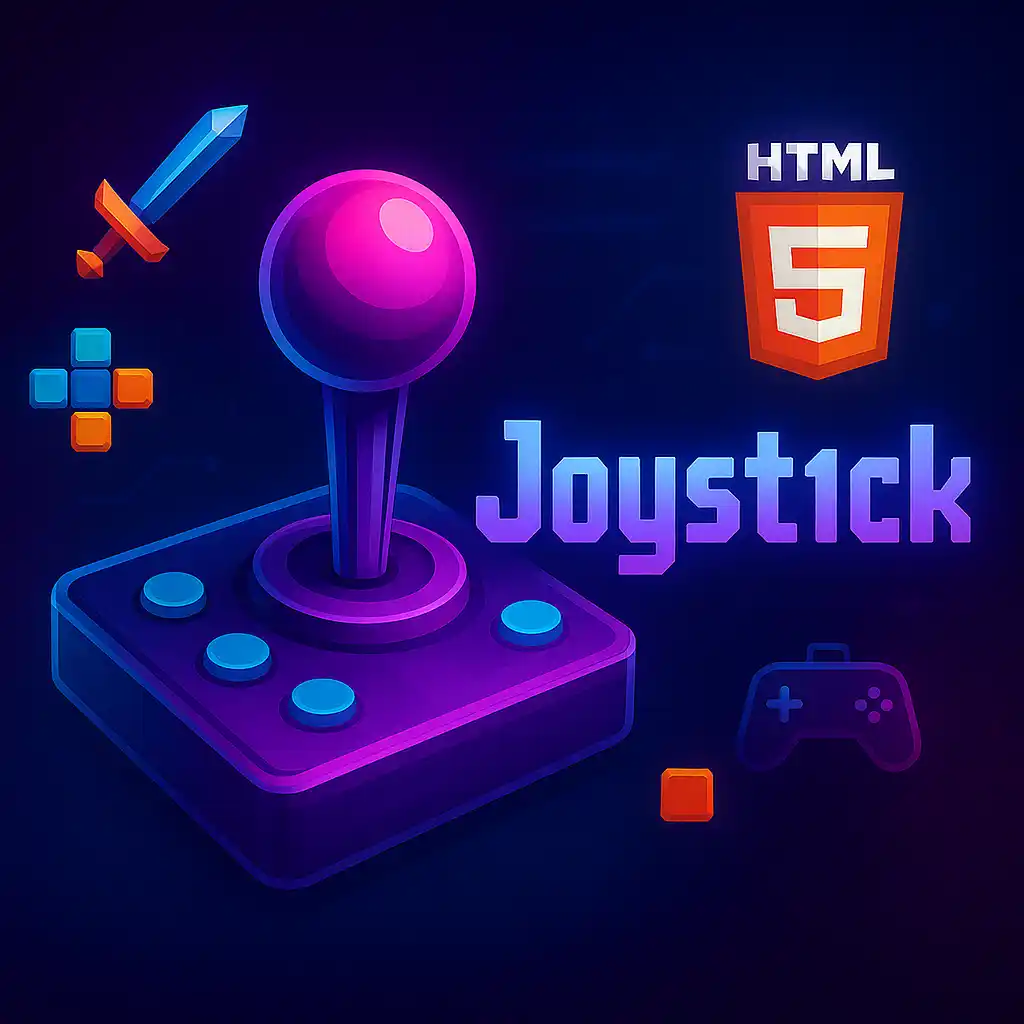