Table of Contents
Implementing a Clipboard Manager in Android Games for Sharing High Scores
Overview
To allow players to copy their high scores to the clipboard and share them from within your Android game, you need to leverage Android’s ClipboardManager. This framework allows easy copy-paste functionality for text and complex data types.
Setting Up ClipboardManager
First, ensure you have access to the Android SDK in your project. Then, you can utilize Android’s Clipboard API as follows:
Play free games on Playgama.com
import android.content.ClipData;
import android.content.ClipboardManager;
import android.content.Context;
public void copyHighScoreToClipboard(Context context, String highScore) {
ClipboardManager clipboard = (ClipboardManager) context.getSystemService(Context.CLIPBOARD_SERVICE);
ClipData clip = ClipData.newPlainText("High Score", highScore);
clipboard.setPrimaryClip(clip);
}
Security Considerations
Ensure that your game adheres to secure clipboard handling practices to protect user data. Always request permission and inform users before accessing the clipboard.
Handling Clipboard on Android 10 and Above
On Android 10 and above, clipboard access is restricted for background apps to enhance privacy. Your game needs to be in the foreground to copy data. Use proper event handling to ensure this functionality executes when the game is actively played:
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.Q) {
// Ensure your app has focus
// Handle clipboard operations here
}
Sharing High Scores
After copying the score to the clipboard, players can paste it anywhere they wish to share. You can optionally integrate a direct share feature using Android’s share intents to create a more seamless experience:
Intent shareIntent = new Intent(Intent.ACTION_SEND);
shareIntent.setType("text/plain");
shareIntent.putExtra(Intent.EXTRA_TEXT, highScore);
startActivity(Intent.createChooser(shareIntent, "Share your high score!"));
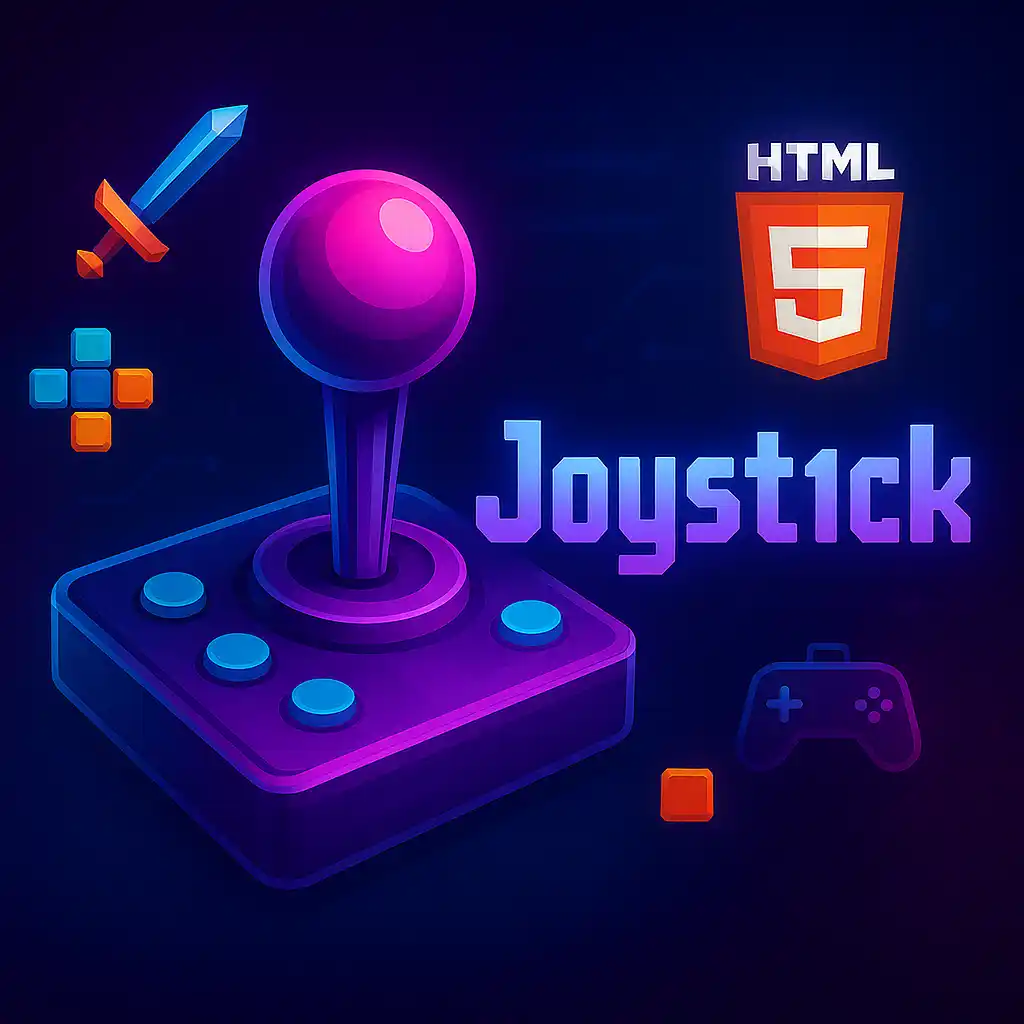