Table of Contents
Drawing an Ellipse for UI Elements in Unreal Engine
Introduction
Drawing custom shapes such as ellipses can enhance the visual design of your game UI. In Unreal Engine, this can be achieved through various techniques, leveraging both Blueprint scripting and C++ capabilities.
Using Blueprint Scripting
Blueprints in Unreal Engine provide a visual scripting method to handle graphical tasks, including drawing shapes:
Play free games on Playgama.com
- Material Function: Create a material that uses a custom UV function to render an ellipse shape. This material can then be applied to UI elements like Image widgets in UMG.
- Canvas Render Target: Use a Canvas Render Target 2D and draw an ellipse using the Draw Line and Draw Polygon methods. You can then map this render target to a UI element.
Implementing in C++
If you’re comfortable with C++, you have more direct control over graphics rendering in Unreal Engine:
- Custom Slate Widget: Create a custom Slate widget by overriding the
OnPaint
function. Use drawing methods likeFCanvas::DrawTile
orFSlateDrawElement::MakeBox
to draw an ellipse. - Procedural Mesh Component: Although mainly for 3D models, a Procedural Mesh Component can be adapted to construct and render a 2D ellipse by defining vertex positions programmatically.
Ellipse Drawing Algorithm
At the core, the Midpoint Ellipse Algorithm can be used for precise ellipse rendering:
void DrawEllipse(FVector2D Center, float Rx, float Ry) { for(float i = 0; i < 360; i += 1) { float Rad = FMath::DegreesToRadians(i); float X = Center.X + Rx * FMath::Cos(Rad); float Y = Center.Y + Ry * FMath::Sin(Rad); // Use Unreal Engine's Drawing API to plot (X, Y) } }
Performance Considerations
- Keep the number of segments in ellipse drawing to a minimum required for smooth appearance to reduce computational overhead.
- Cache calculated ellipse shapes when possible to avoid redundancy and improve performance.
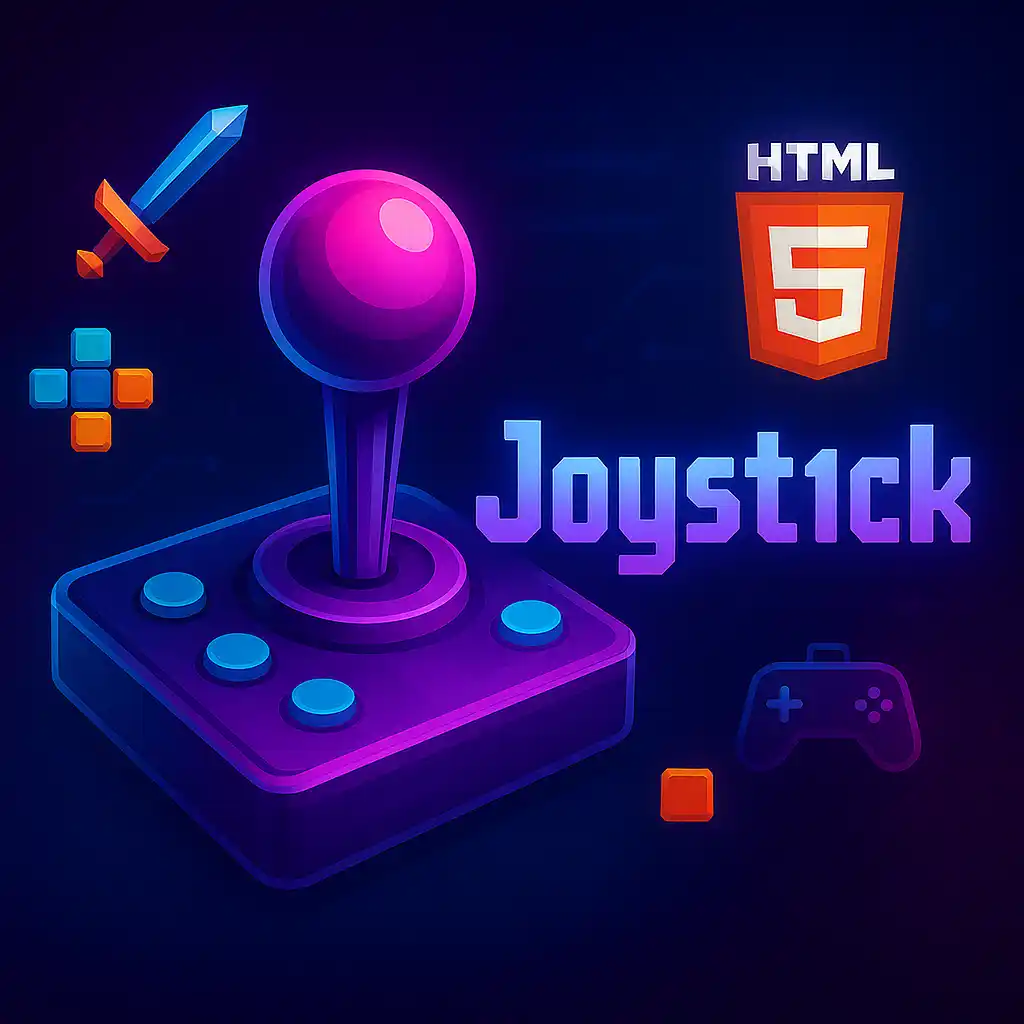