Table of Contents
Implementing Acceleration in Unity
Understanding Acceleration
Acceleration in game development refers to the rate of change of velocity of an object over time. Typically, an object’s acceleration can be calculated using the formula: Acceleration = (Final Velocity - Initial Velocity) / Time
.
Using Distance and Time
To calculate acceleration using distance and time, you can utilize the kinematic equation: Final Velocity2 = Initial Velocity2 + 2 * Acceleration * Distance
. Rearranging for acceleration gives: Acceleration = (Final Velocity2 - Initial Velocity2) / (2 * Distance)
. If the initial velocity is zero (common for starting movements), this simplifies further.
Play free games on Playgama.com
Implementing in Unity
- Initialize Variables: Define initial velocity, final velocity, time, and distance for your object.
- Calculate Acceleration: Use the kinematic equations above or Unity’s physics engine for realistic computations.
float initialVelocity = 0f; // Assuming object starts at rest float finalVelocity = 10f; // Example target speed in units per second float distance = 50f; // Distance object needs to cover float time = 5f; // Time duration in seconds float acceleration = (finalVelocity - initialVelocity) / time; // Adjusting formula if distance is provided // acceleration = (Mathf.Pow(finalVelocity, 2) - Mathf.Pow(initialVelocity, 2)) / (2 * distance);
- Apply Acceleration: In the
Update
orFixedUpdate
methods, apply the calculated acceleration to the object’s velocity.void Update() { float currentVelocity = initialVelocity + acceleration * Time.deltaTime; transform.position += transform.forward * currentVelocity * Time.deltaTime; initialVelocity = currentVelocity; // Update for the next frame }
- Tuning and Physics: Use Unity’s Rigidbody component if dynamic physics interactions are required. This allows for automatic calculation of movements under forces and inertia.
Best Practices
- Utilize Unity’s Rigidbody for physics-based movement for realistic interactions.
- Use
Time.deltaTime
to ensure frame-rate independent calculations. - Test and adjust parameters like initial velocity and distance to suit gameplay feel and responsiveness.
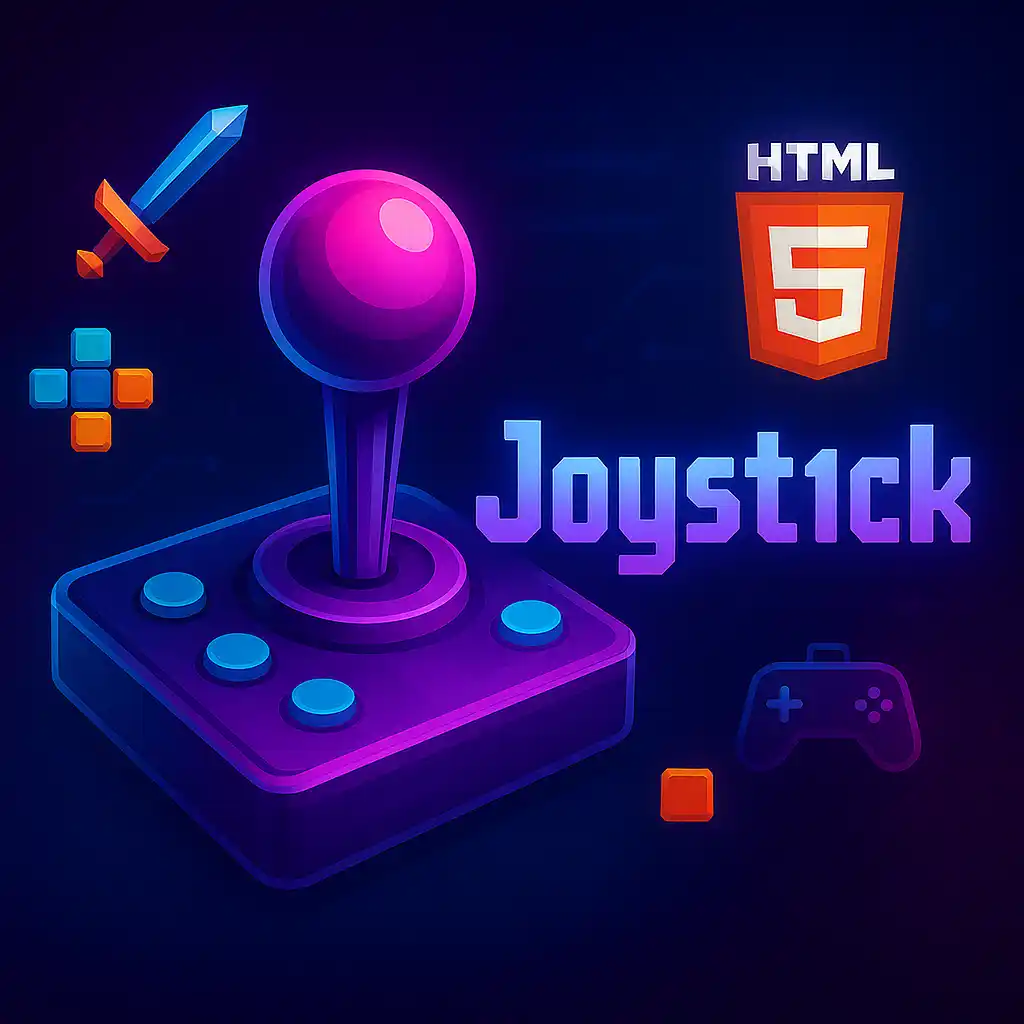