Table of Contents
Implementing a Fullscreen Toggle in Unity
Using Screen Class
In Unity, you can easily toggle fullscreen mode using the Screen
class. Here’s a simple way to implement this feature:
void ToggleFullscreen() {
Screen.fullScreen = !Screen.fullScreen;
}
Attach this method to a button or key press event to allow players to switch between fullscreen and windowed mode.
Play free games on Playgama.com
Using Player Preferences
To save the player’s preference for fullscreen mode, use the PlayerPrefs
class:
void Start() {
bool isFullScreen = PlayerPrefs.GetInt("Fullscreen", 1) == 1;
Screen.fullScreen = isFullScreen;
}
Additionally, set the value when changing fullscreen mode:
void ToggleFullscreen() {
Screen.fullScreen = !Screen.fullScreen;
PlayerPrefs.SetInt("Fullscreen", Screen.fullScreen ? 1 : 0);
}
Implementing a Fullscreen Toggle in Unreal Engine
Using C++
In Unreal Engine, you can modify the fullscreen mode directly within a C++ class. Use the following code to toggle fullscreen mode:
void ToggleFullscreen() {
if (GEngine) {
GEngine->GameViewport->GetWindow()->SetWindowMode(EWindowMode::Fullscreen);
}
}
This method will change the window mode to fullscreen. Use EWindowMode::Windowed
to revert to windowed mode.
Using Blueprints
You can also achieve this using Blueprints by using the Execute Console Command
node with the command: "r.setres 1920x1080f"
for fullscreen or "r.setres 800x600w"
for windowed mode.
Best Practices
- Ensure that the resolution is supported by the monitor to avoid exceptions.
- Provide visual feedback to the player on resolution change.
- Store player preferences using a configuration file or in-game settings menu.
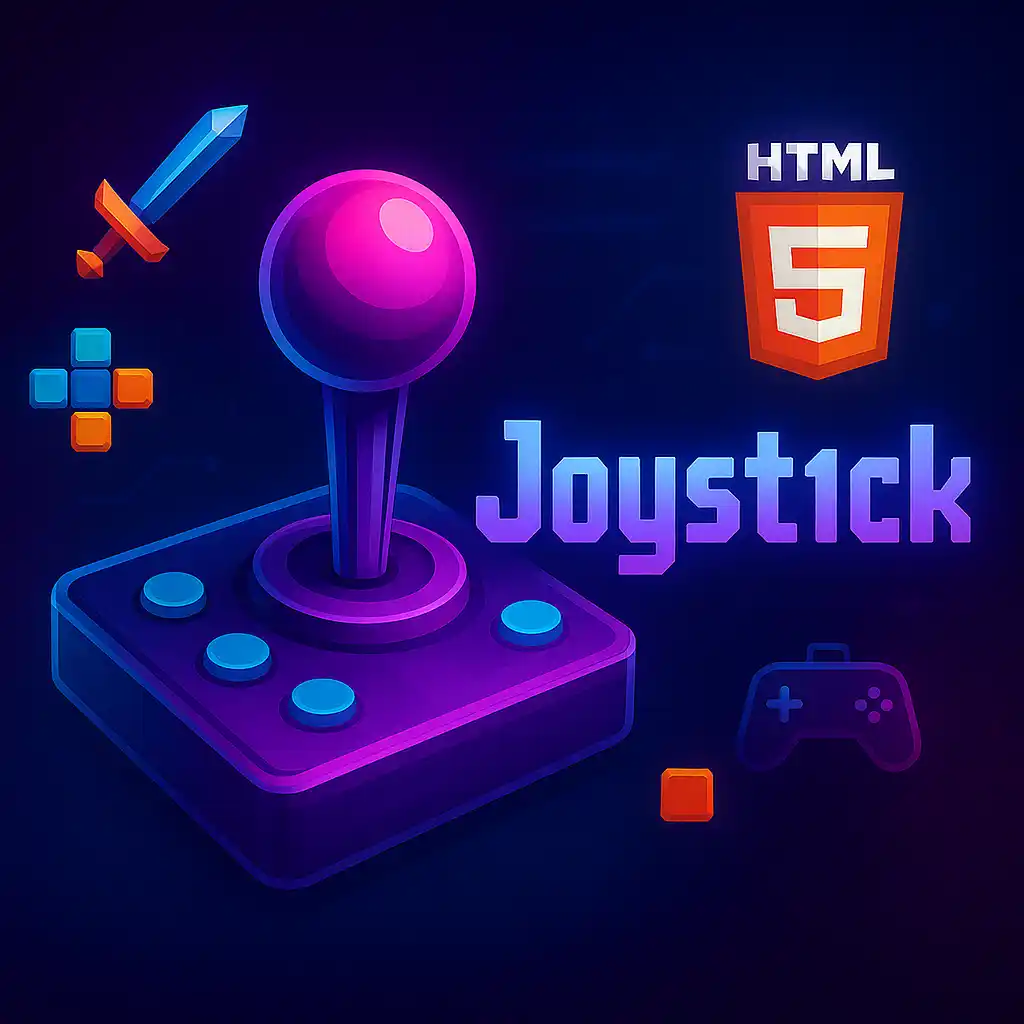