Table of Contents
Setting the Origin Point in a Graph for a Game’s User Interface
Properly configuring the origin point in a game’s user interface graph is crucial for accurate data visualization and user interpretation. Here are the steps to achieve this in Unity:
1. Understanding the Coordinate System
Unity uses a coordinate system where the origin, (0,0,0), can be adjusted to suit your application needs. This flexibility is essential in setting up efficient and accurate data visualizations in game UI.
Play free games on Playgama.com
2. Adjusting the Graph’s Origin Point
- Create a GameObject: Start by creating a new GameObject in your UI canvas that will represent your graph’s base point.
- Attach a Script: Write a custom script to manage the graph’s origin. The script should define the origin point based on the desired layout, possibly by setting the transform position of the GameObject.
- Transform Origin: Use Unity’s
Transform
component to adjust the position, and ensure that all data points or UI elements are calculated with respect to this origin. This will require recalculating positions relative to this new origin point.
3. Using Floating Origin Techniques
If your graph is part of a larger dynamic environment, consider employing a floating origin approach. This can help maintain numerical precision and performance, especially in large-scale data visualization, where the graph may need to dynamically update or transform without losing fidelity.
4. Practical Implementation
public class GraphOrigin : MonoBehaviour {
public Vector3 customOrigin = Vector3.zero;
void Start() {
Transform graphTransform = GetComponent<Transform>();
graphTransform.position = customOrigin;
// Additional code to reposition and rescale data points accordingly
}
}
5. Testing and Optimization
Once the origin point is established, ensure that all data trends and visual elements are correctly aligned. Test various data scenarios to confirm the functionality. Consider using Unity’s Profiler to optimize performance, especially if your graph updates frequently and dynamically.
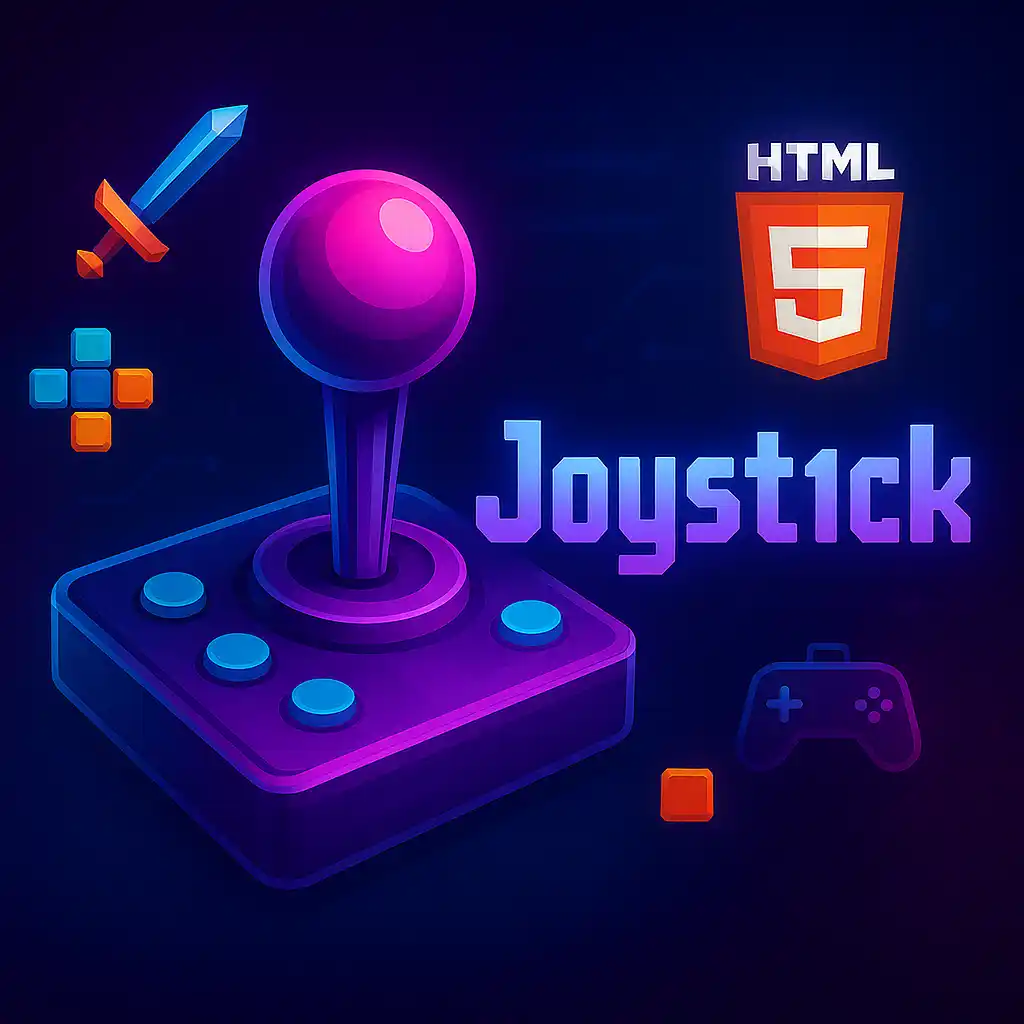