Table of Contents
Troubleshooting Input Handling for the Backspace Key in Unity’s UI
When developing UI text fields in Unity, encountering issues where the backspace key fails to respond correctly can disrupt user experience. Here are steps to troubleshoot and resolve this input handling issue:
1. Check Input Settings
- Navigate to Unity Preferences → Input Manager and ensure that the backspace key is mapped correctly. Confirm that it is not in conflict with other input settings within the game.
2. Inspect Event System
- Ensure your scene contains a properly configured
EventSystem
component. This system is responsible for capturing input events, and its absence or misconfiguration might lead to input issues. Verify theStandaloneInputModule
settings, which typically manages keyboard input.
3. Debugging Backspace Logic
- Review your code handling text input. If manipulating strings directly, ensure that your backspace logic accurately modifies the string as expected. For example, consider using
string.Remove
to correctly handle character deletion:
// C# snippet for handling backspace
if (Input.GetKeyDown(KeyCode.Backspace) && myTextField.text.Length > 0) {
myTextField.text = myTextField.text.Remove(myTextField.text.Length - 1);
}
4. Test on Different Platforms
- If possible, test the issue across different platforms. Sometimes, the problem could stem from specific hardware or platform settings. Troubleshoot accordingly by checking platform-specific input handling configurations.
5. External Libraries or Packages
- Identify any third-party libraries or UI frameworks in use which might override default input behaviors. Temporarily disabling these libraries can determine if they are the source of conflicts.
By following these steps, developers can troubleshoot and potentially resolve issues with the backspace key not responding correctly, ensuring a better user interaction in Unity’s UI text fields.
Play free games on Playgama.com
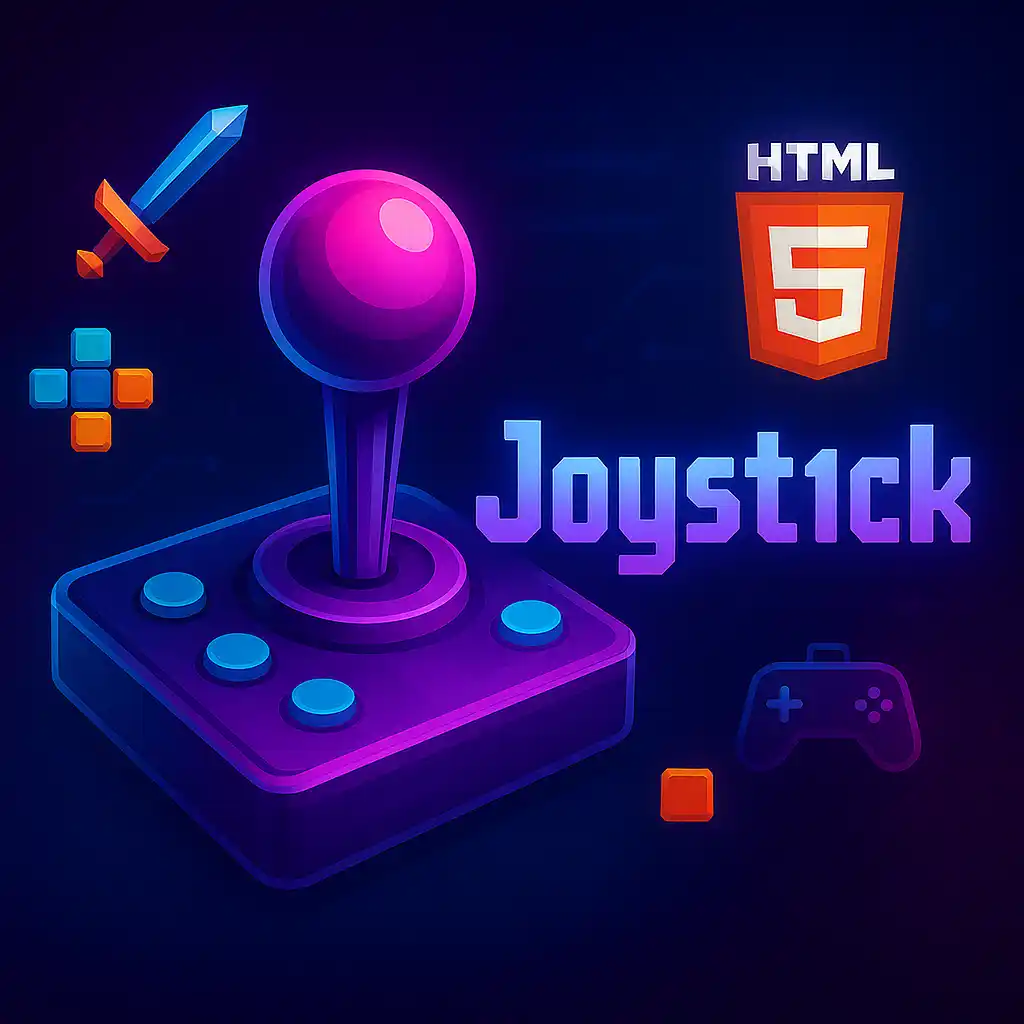