Table of Contents
Calculating Total Distance Traveled by an NPC Using Waypoints in Unity
In Unity, calculating the total distance traveled by a non-player character (NPC) using waypoints involves iterating through a list of predefined waypoints and summing the Euclidean distances between consecutive points as the NPC moves along these paths.
Play free games on Playgama.com
Step-by-Step Implementation
- Setup Waypoints: Create a list of waypoint
Vector3
objects representing the NPC’s path. - Initialize Variables: Initialize a float variable to keep track of the total distance traveled, e.g.,
float totalDistance = 0f;
. - Iterate Through Waypoints: For each pair of consecutive waypoints, compute the distance and add it to the total distance. Here’s a Unity C# script example:
using UnityEngine; using System.Collections.Generic; public class DistanceCalculator : MonoBehaviour { public List<Vector3> waypoints; private float totalDistance; void Start() { totalDistance = CalculateTotalDistance(waypoints); Debug.Log("Total Distance Traveled: " + totalDistance); } float CalculateTotalDistance(List<Vector3> points) { float distance = 0f; for (int i = 0; i < points.Count - 1; i++) { distance += Vector3.Distance(points[i], points[i + 1]); } return distance; } }
Considerations
- Dynamic Paths: If your NPC’s path changes dynamically, ensure to recalculate the distance regularly.
- Smoothing: Consider interpolating between waypoints for smoother traversal, which may require a more complex distance calculation.
Optimization Tips
- Use Cached Results: If waypoints do not change, cache the results to avoid redundant calculations.
- Physics Updates: Consider using
FixedUpdate()
for consistent results during physics calculations.
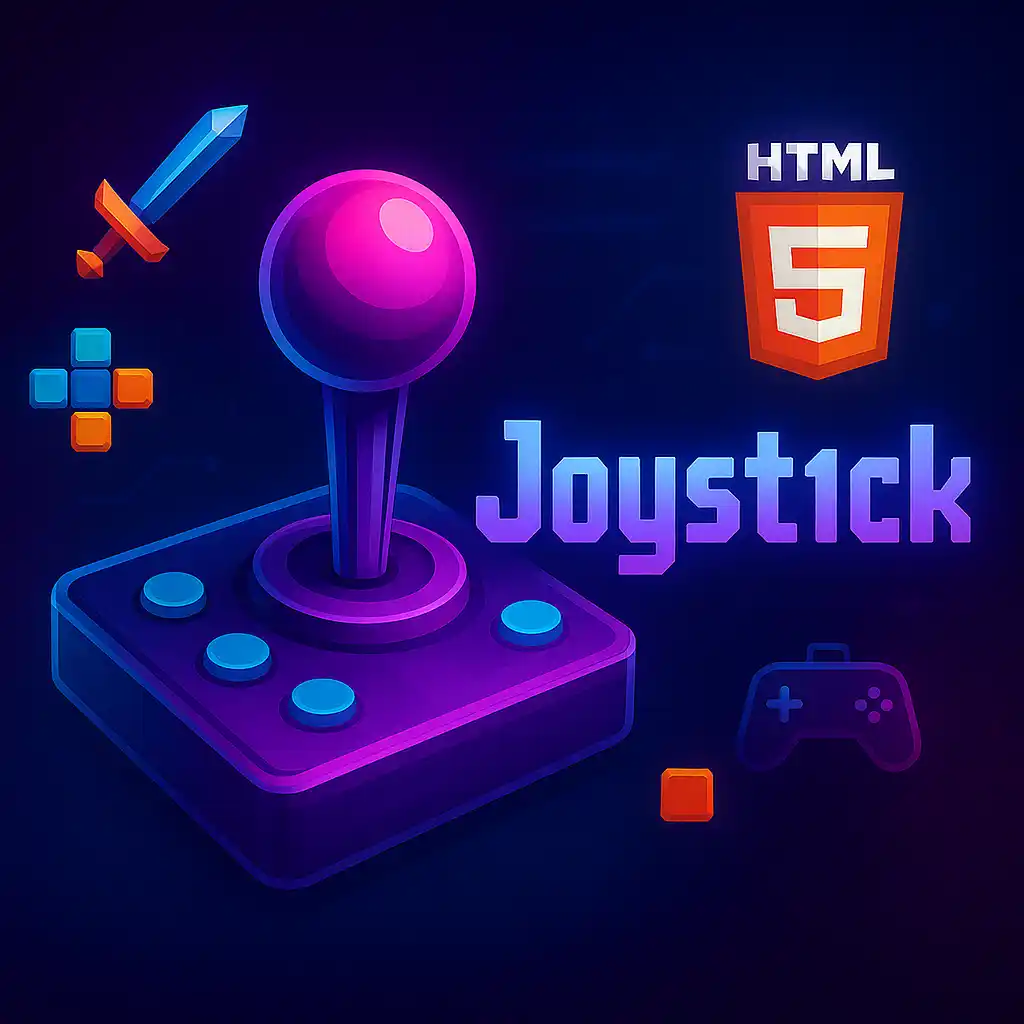