Table of Contents
Integrating a Whisper Chat Feature in Unity
To integrate a whisper chat feature akin to Twitch in your multiplayer game using Unity, you will need to establish a secure and efficient communication protocol between client and server. Here’s how you can do this:
1. Set Up the Server
Firstly, ensure you have a dedicated server to manage connections and handle routing for whisper messages. You can use an existing solution like Photon Engine or set up your own using WebSockets for real-time data exchange.
Play free games on Playgama.com
2. Define the Communication Protocol
Design a protocol for sending and receiving messages. A typical message might include:
{ "type": "whisper", "sender": "Player1", "receiver": "Player2", "message": "Hello!" }
This JSON format is lightweight and easy to parse.
3. Implement Client-Side Whisper Logic
In Unity, utilize C# and the Unity networking API to implement the client-side logic:
- Connect to the server using a WebSocket connection.
- Listen for incoming messages and filter ‘whisper’ types specifically.
- Display messages in an isolated chat UI component.
4. Ensure Secure Communication
Use SSL/TLS encryption for your WebSocket connections to ensure the privacy of player communications. You can achieve this with readily available libraries like websocket-sharp
which supports wss:// protocols.
5. Optimize for Performance
- Batch message retrieval to minimize server calls.
- Use compression techniques to reduce the size of data packets.
Example Code: WebSocket Connection in Unity
using WebSocketSharp; public class ChatClient : MonoBehaviour { private WebSocket ws; void Start() { ws = new WebSocket("wss://yourserver.com"); ws.OnMessage += (sender, e) => Debug.Log("Received: " + e.Data); ws.Connect(); } void SendWhisper(string recipient, string message) { ws.Send(JsonUtility.ToJson(new { type = "whisper", sender = "YourPlayerName", receiver = recipient, message = message })); } }
This example demonstrates how to establish a WebSocket connection and send whisper messages. Adjust the code to fit your architecture and error handling practices.
By following these steps and tweaking them to meet your game’s specific requirements, you can successfully integrate a whisper chat feature similar to Twitch, thus enhancing the player communication experience in your multiplayer game.
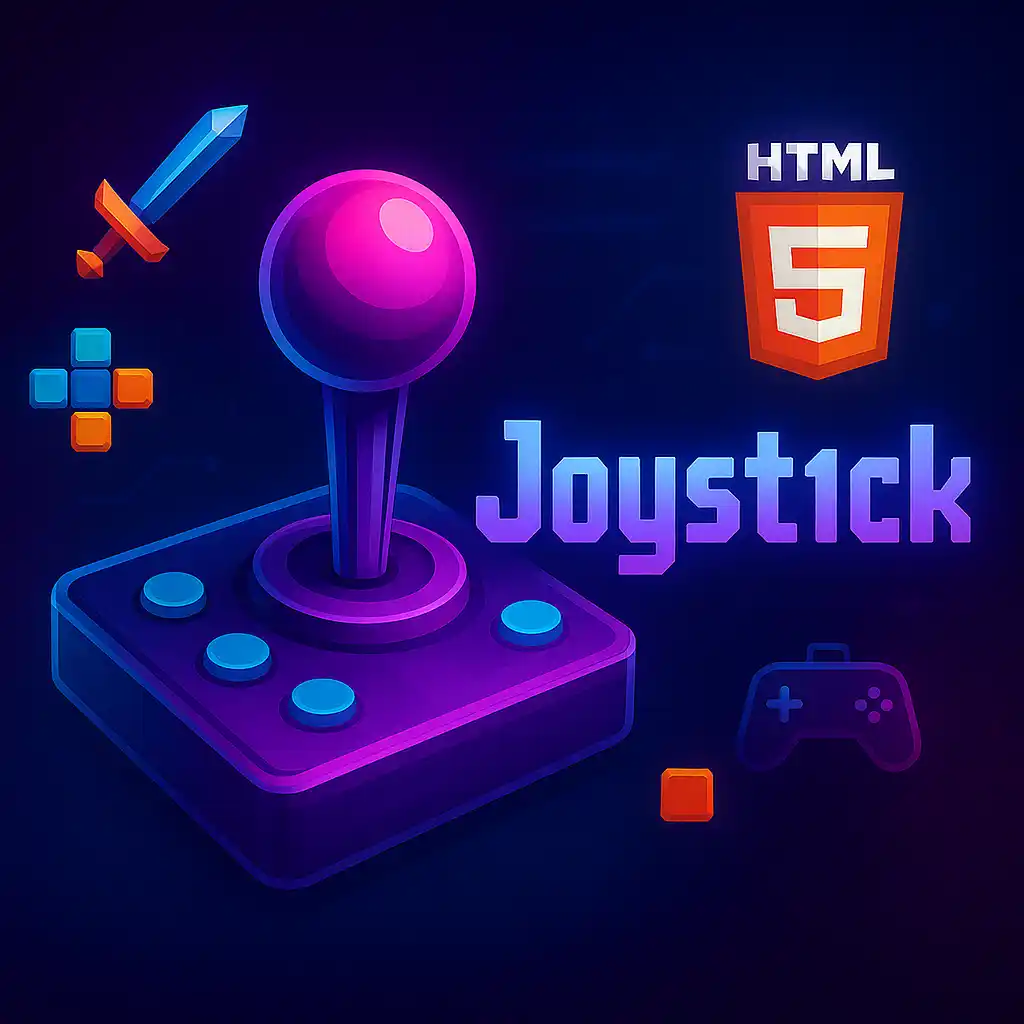