Table of Contents
Implementing a Triangular Mesh in Unity
Understanding Meshes
In Unity, a mesh is essentially a collection of vertices, edges, and faces used to define the shape of a 3D object. To implement a triangular mesh, you’ll need to define these elements properly.
Creating a Triangular Mesh
- Vertices: Define an array of Vector3 points that represents the corners of your triangles.
- Triangles: Specify the order in which the vertices should connect to form triangles. Each triangle is represented by three consecutive indices from the vertices array.
- Normals: For shading and lighting, normals must be defined for each vertex. Use Vector3 to specify direction.
- UVs: Define texture coordinates to map textures to your mesh.
using UnityEngine;public class CreateMesh : MonoBehaviour { void Start() { Mesh mesh = new Mesh(); Vector3[] vertices = new Vector3[] { new Vector3(0, 0, 0), new Vector3(1, 0, 0), new Vector3(0, 1, 0), new Vector3(0, 0, 1) }; int[] triangles = new int[] { 0, 2, 1, 0, 3, 2 }; Vector3[] normals = new Vector3[vertices.Length]; for (int i = 0; i < normals.Length; i++) normals[i] = Vector3.up; Vector2[] uv = new Vector2[] { new Vector2(0, 0), new Vector2(1, 0), new Vector2(0, 1), new Vector2(0, 0) }; mesh.vertices = vertices; mesh.triangles = triangles; mesh.normals = normals; mesh.uv = uv; GetComponent().mesh = mesh; }}
Adding Collision Shapes
Using Unity’s Built-in Colliders
Unity provides built-in colliders such as box, sphere, and capsule colliders. To match a triangle shape, it’s more common to use MeshCollider, but be aware of the performance implications.
Play free games on Playgama.com
- Mesh Collider: Attach this to use the custom mesh for collision detection.
gameObject.AddComponent
Optimizing Collision Detection
Use convex meshes when possible. For non-convex shapes, consider performance costs as they are computationally expensive.
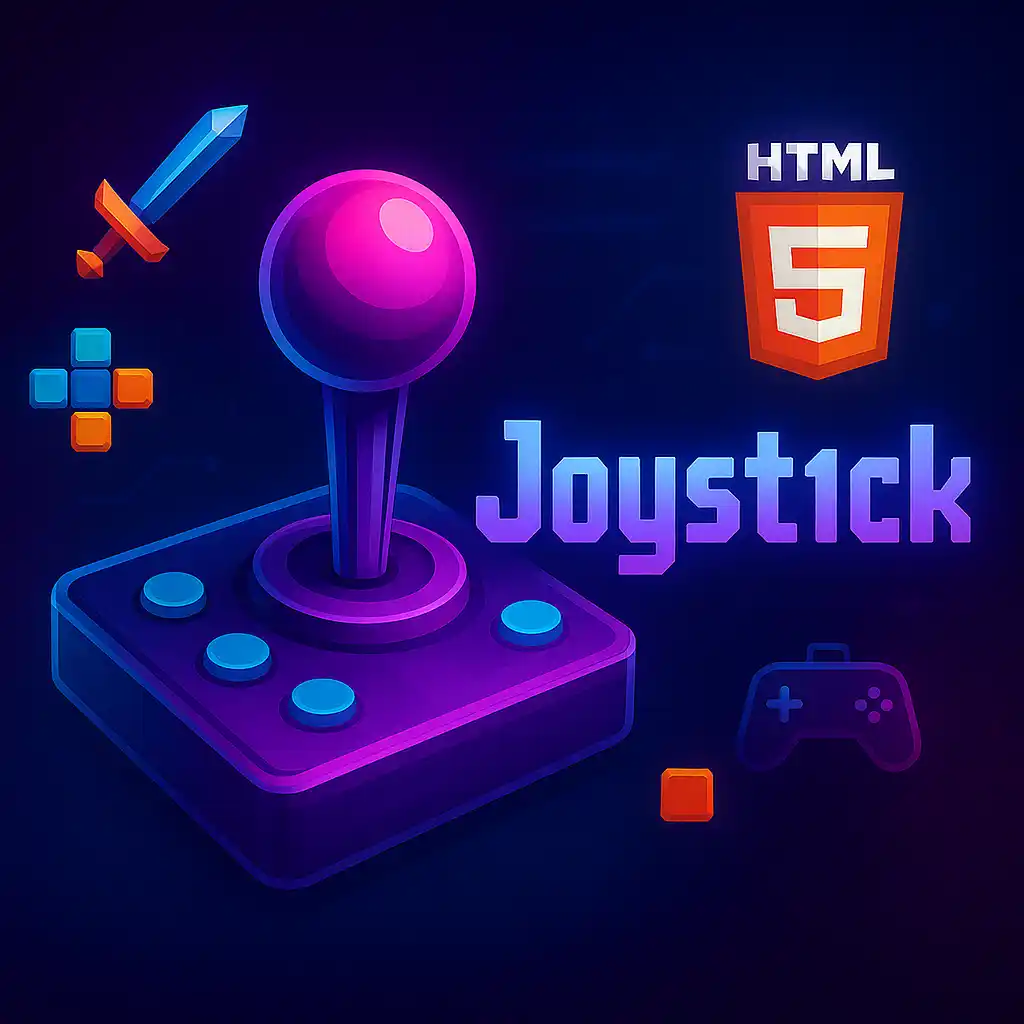