Table of Contents
Implementing a Score Rounding Function in Unity
To round up player scores to the nearest ten in Unity, you can implement a simple function within your script handling leaderboard operations. This ensures consistency in score presentation and facilitates easier score comparisons.
Step-by-Step Guide
Follow this approach to implement a score rounding function:
Play free games on Playgama.com
- Import Necessary Namespaces: Ensure you have access to necessary Unity namespaces such as
System
. This is generally available by default in Unity scripts. - Create the Rounding Function: Write a function that takes an integer score and applies the mathematical operation to round it up to the nearest ten. Here’s a simple implementation:
using UnityEngine;
public class LeaderboardManager : MonoBehaviour
{
public int RoundUpScore(int score)
{
return ((score + 9) / 10) * 10;
}
}
- Integrate with Leaderboard Logic: Use this function when storing or displaying player scores. Ensure to call this within your updating logic, like so:
int playerScore = 87; // Example score
int roundedScore = RoundUpScore(playerScore);
Debug.Log("Rounded Score: " + roundedScore); // Outputs: 90
Explanation of the Rounding Logic
- The expression
(score + 9) / 10
effectively rounds scores up by first adding 9 and then integer division by 10, which truncates the decimal, effectively achieving rounding up to the nearest 10. - Multiplying by 10 after truncation adjusts the result back into tens.
Practical Considerations
- Performance: This computation is efficient for most scenarios with negligible performance impact.
- Edge Cases: Handle scenarios where the score might be negative or extremely high, ensuring the game logic accommodates all possibilities.
Testing
After implementation, thoroughly test this rounding function in various scenarios to ensure reliable behavior across different score values.
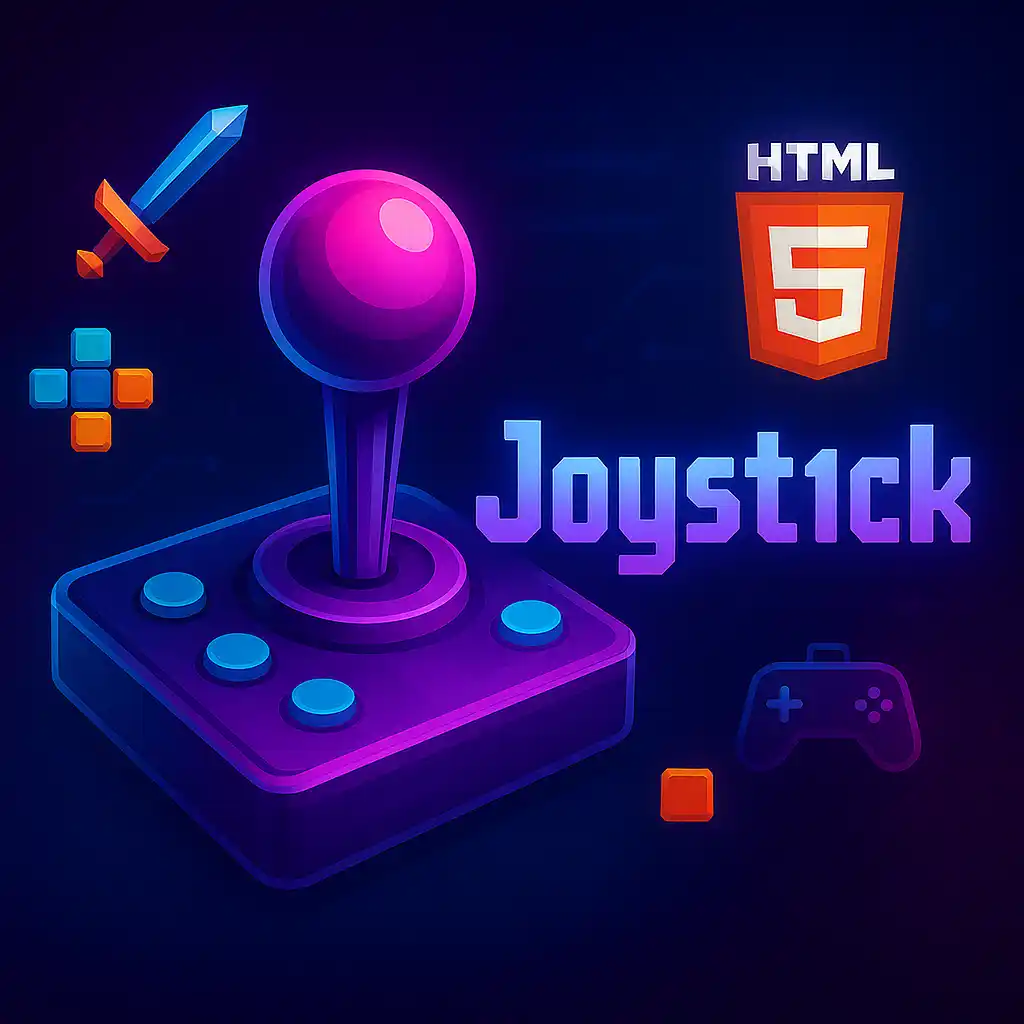