Table of Contents
Implementing Rounding Functions in Unity
Accurate display of decimal values is crucial in providing clear game statistics. In Unity, you can manage and manipulate decimal values effectively using C#. Below, we outline a step-by-step approach to implement a rounding function for decimal values:
Using Mathf.Round
and Custom Methods
Unity’s Mathf Library: Unity provides Mathf.Round
for rounding float numbers. However, to specifically round to two decimal places, you need to implement a custom function:
Play free games on Playgama.com
public static float RoundToTwoDecimalPlaces(float value) {
return Mathf.Round(value * 100f) / 100f;
}
This method utilizes Mathf.Round
to round the number value effectively to two decimal places by employing multiplication and division to shift the decimal.
Implementing Decimal Precision for Game Stats
- Displaying In-Game Currency and Stats: Ensure currency values and game stats are presented in a user-friendly manner, for instance:
public string GetFormattedStat(float statValue) {
float roundedValue = RoundToTwoDecimalPlaces(statValue);
return roundedValue.ToString("F2"); // Formats with two decimal points
}
- Floating Point Precision: Be cautious of floating-point precision issues that might occur during arithmetic operations, and test rounding functions thoroughly with edge cases.
- Alternative Methods: Consider using
decimal
data type for more precise calculations when displaying sensitive financial game data.
Troubleshooting Rounding in Unity
Inconsistencies or bugs in rounding can arise from:
- Improper Type Handling: Ensure correct data types are used for calculations to prevent overflow or precision loss.
- Complex Calculations: When working with complex mathematical operations, maintain precision throughout all computations before applying rounding functions.
To address these issues, regular testing and debugging using Unity’s profiler or logging is recommended.
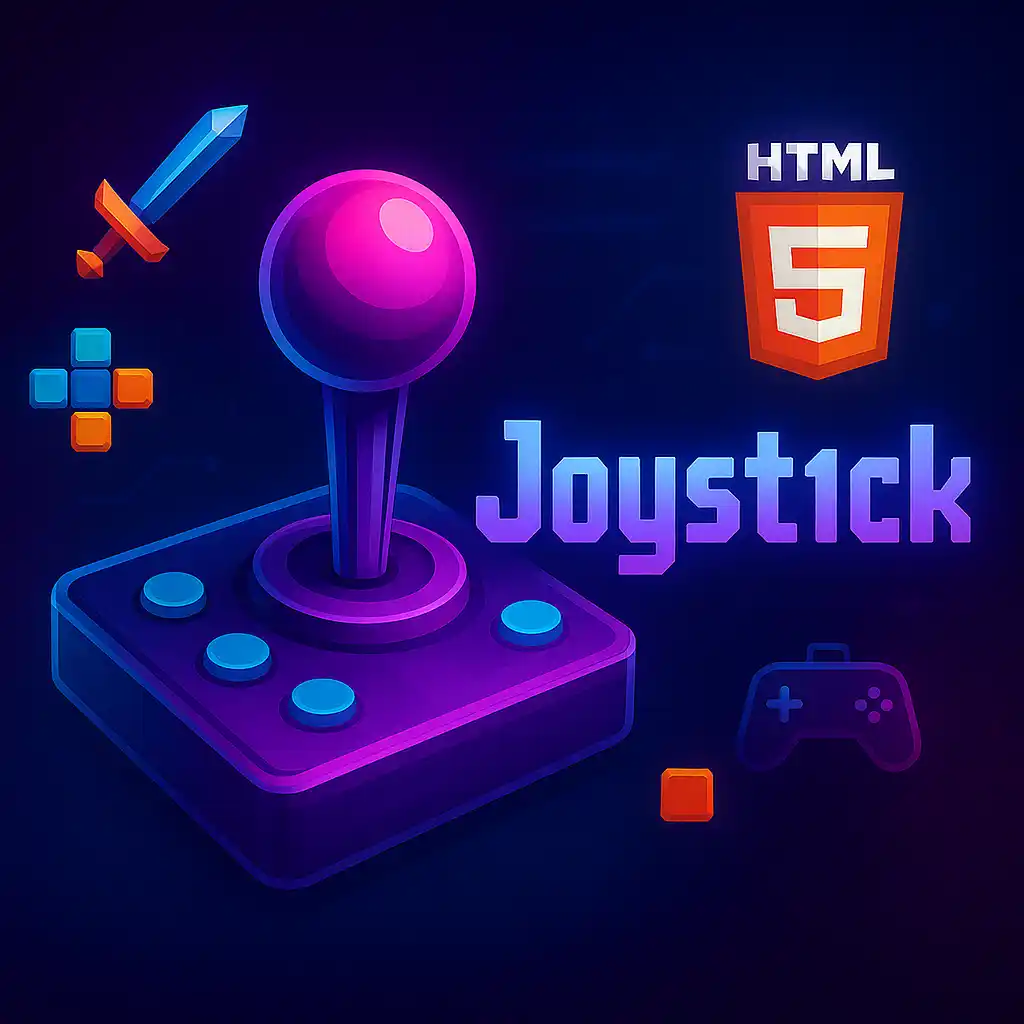