Table of Contents
Preventing Division by Zero and Ensuring Stability in Unity
Understanding Division by Zero in Game Development
In game development, especially when working with physics or mathematical computations, division by zero can lead to critical errors, causing the game to crash or behave unexpectedly. Ensuring stability in such calculations is crucial for a robust gaming experience.
Implementing Checks in Calculations
To prevent division by zero, it is essential to implement conditional checks. Here’s a basic example in C# for Unity:
Play free games on Playgama.com
float divide(float numerator, float denominator) { if (Mathf.Approximately(denominator, 0.0f)) { Debug.LogWarning("Attempted division by zero"); return 0; } return numerator / denominator; }
This function uses Mathf.Approximately
to avoid issues with floating-point precision, offering a reliable way to check if a number is effectively zero.
Mathematical Stability Techniques
- Clamp Values: Use techniques like clamping values to a minimum threshold before division. This ensures that the denominator never reaches zero.
- Normalization: When handling vectors or other mathematical entities, normalize them to avoid scenarios where the length or magnitude could be zero.
- Safe Defaults: Design algorithms that revert to safe default values when singularities are detected.
Leveraging Unity’s Debugging Tools
Utilize Unity’s logging system to log warnings or errors when division by zero situations are detected. This can help identify problematic code during development:
if (denominator == 0) { Debug.LogError("Division by zero detected in method XYZ"); }
Ensuring Comprehensive Testing
Finally, comprehensive testing is vital. Employ automated tests to simulate scenarios where division by zero might occur. Unity Test Framework can be beneficial for creating unit tests that ensure your calculations handle edge cases safely.
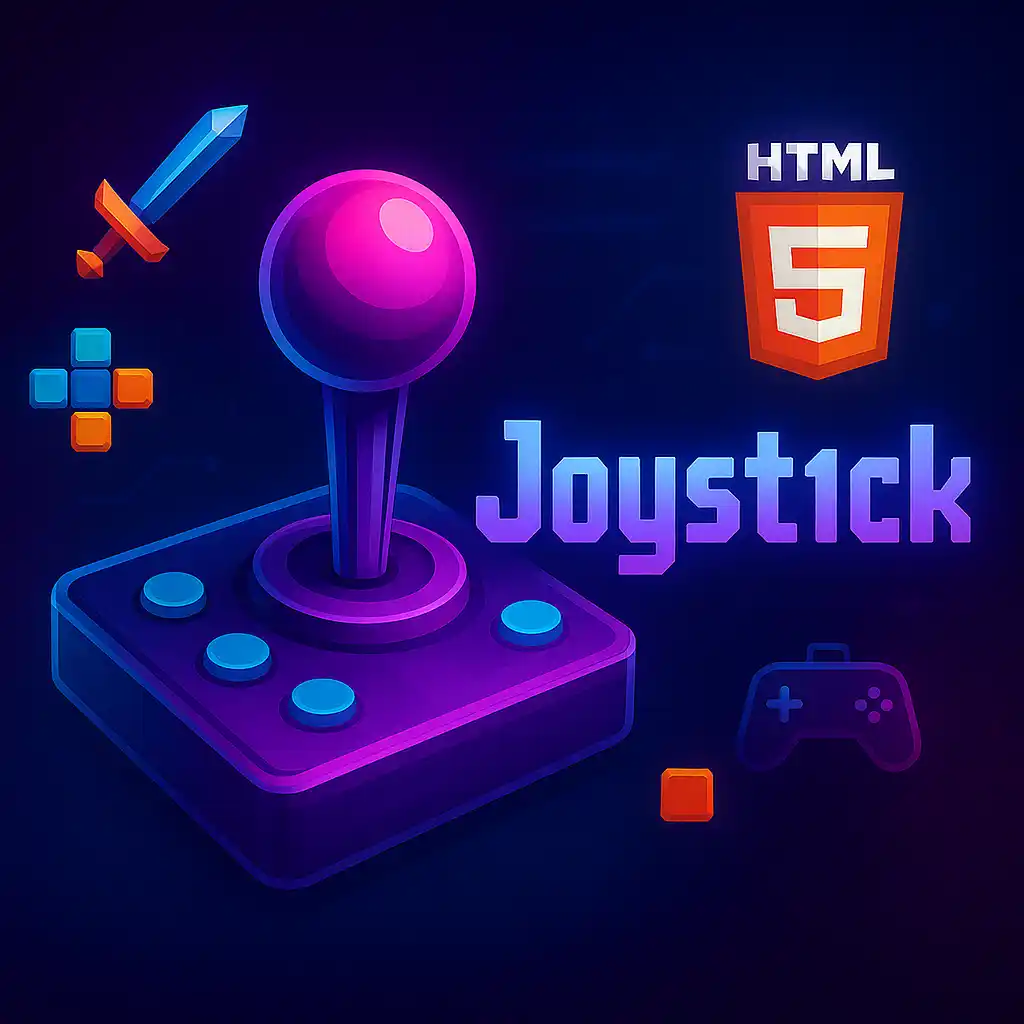