Table of Contents
Simulating Drag Force in Unity
To accurately simulate drag force in Unity and enhance the realism of your racing game, it’s important to understand the physics behind drag force and how to implement it effectively in the game environment.
Understanding Drag Force
Drag force is a resistance force caused by the motion of a body through a fluid, such as air. It can be modeled by the equation:
Play free games on Playgama.com
Fd = 0.5 * Cd * ρ * A * v2
- Fd: Drag force.
- Cd: Drag coefficient, which depends on the shape of the object.
- ρ: Density of the fluid (air).
- A: Reference area of the object.
- v: Velocity of the object.
Implementing in Unity
- Physics Setup: Use Unity’s Rigidbody component to enable physics on your car objects. Ensure that the
mass
anddrag
properties are set appropriately. - Real-Time Calculations: Implement a script to compute the drag force in real-time. Here’s a simple example:
using UnityEngine;
public class DragSimulation : MonoBehaviour
{
public float dragCoefficient = 0.47f; // Example for a sphere
public float referenceArea = 1.0f; // Adjust based on your object's size
private Rigidbody rb;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void FixedUpdate()
{
Vector3 velocity = rb.velocity;
float speed = velocity.magnitude;
float dragForceMagnitude = 0.5f * dragCoefficient * AirDensity() * referenceArea * speed * speed;
Vector3 dragForce = -dragForceMagnitude * velocity.normalized;
rb.AddForce(dragForce);
}
float AirDensity()
{
return 1.225f; // Average air density at sea level in kg/m³
}
}
- Tuning Parameters: Adjust the
dragCoefficient
andreferenceArea
to match the physical characteristics of your vehicles, considering factors like vehicle shape and size. - Performance Optimization: Use approximation methods or simplify calculations for lower-end hardware, if necessary, to maintain performance.
LSI Integration
Incorporating LSI (Latent Semantic Indexing) techniques, such as physics-based game dynamics and mathematical modeling of forces, can further refine your simulation approach. These strategies ensure that your game leverages sophisticated and realistic simulations, maintaining both performance and authenticity.
Testing and Validation
Once implemented, it is crucial to test the drag behavior under various conditions to validate its realism and adjust the coefficients as required. Utilizing the Unity Profiler can help verify performance efficiency and dynamics accuracy during gameplay.
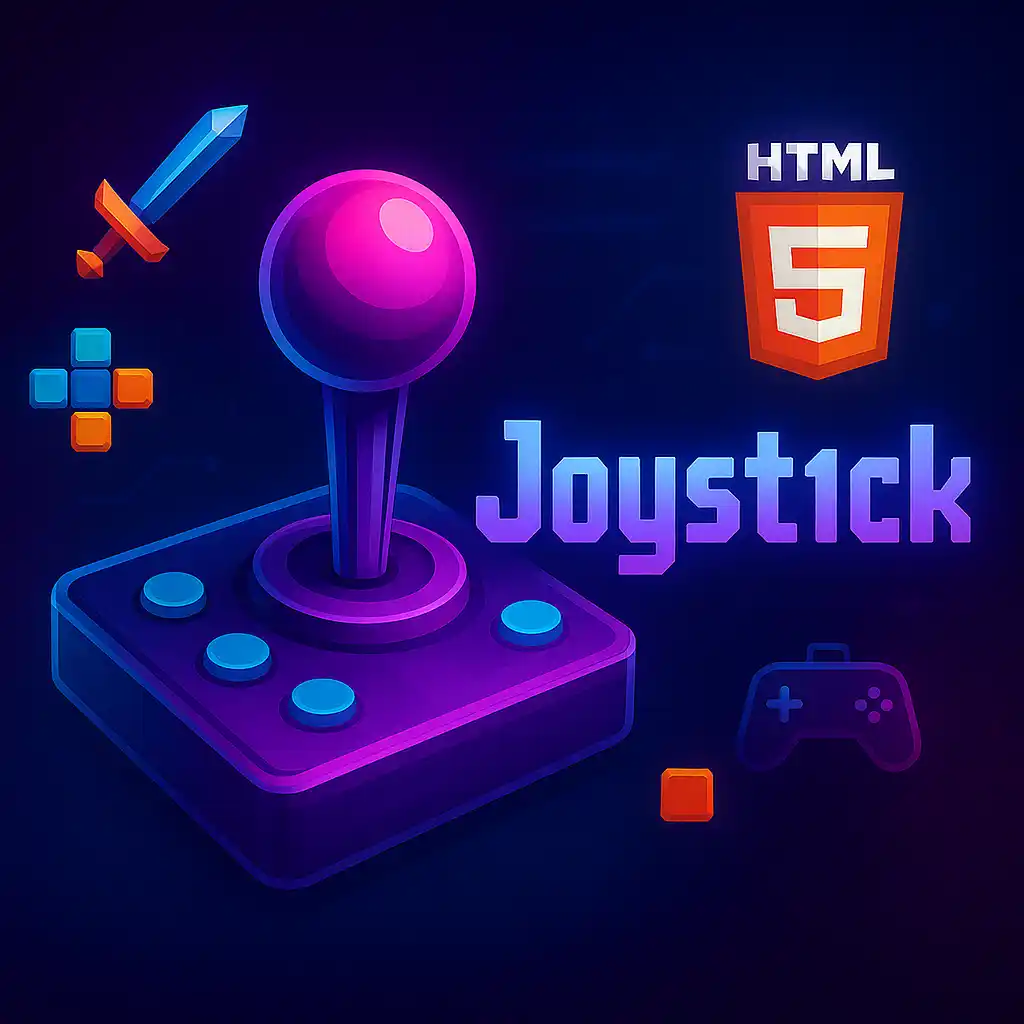