Table of Contents
Drawing a 3D Cone Mesh Programmatically in Godot
Creating a 3D cone mesh programmatically in Godot involves using the built-in MeshInstance
and ArrayMesh
classes. Below are the steps you can follow to achieve this:
1. Define the Cone’s Properties
Firstly, you’ll need to specify the properties of the cone, such as its height, base radius, and the number of segments to approximate the circular base. The segment count will determine the smoothness of the cone.
Play free games on Playgama.com
var height: float = 2.0
var radius: float = 1.0
var segments: int = 36
2. Set Up the Mesh Data
We’ll use arrays to specify the vertices, normals, and indices for the cone. The cone consists of a circular base and a peak joining at the center:
var vertices = []
var indices = []
var normals = []
3. Generate Vertices and Indices
Loop through the segment count to place vertices along the base’s circumference and then add a vertex at the cone’s peak:
for i in range(segments):
var angle = (PI * 2 / segments) * i
var x = radius * cos(angle)
var z = radius * sin(angle)
vertices.append(Vector3(x, 0, z))
if i < segments - 1:
indices.extend([i, i + 1, segments])
else:
indices.extend([i, 0, segments])
# Append the peak vertex
vertices.append(Vector3(0, height, 0))
4. Define Normals and UVs
Normals are essential for correct lighting calculations. Compute them by averaging each triangle's normal:
for i in range(segments):
var normal = Vector3(0, 1, 0).normalized()
normals.append(normal)
UV mapping is optional but can be computed similarly to ensure textures display correctly on the cone's surface.
5. Build the Mesh
Use an ArrayMesh
to store and assemble the data:
var mesh = ArrayMesh.new()
var arrays = []
arrays.resize(Mesh.ARRAY_MAX)
arrays[Mesh.ARRAY_VERTEX] = vertices
arrays[Mesh.ARRAY_NORMAL] = normals
arrays[Mesh.ARRAY_INDEX] = indices
mesh.add_surface_from_arrays(Mesh.PRIMITIVE_TRIANGLES, arrays)
6. Attach to a MeshInstance
Finally, assign the mesh to a MeshInstance
in your scene:
var mesh_instance = MeshInstance.new()
mesh_instance.mesh = mesh
Conclusion
This example demonstrates how to use Godot's scripting capabilities to generate custom geometric shapes programmatically, facilitating dynamic and procedural content in your games.
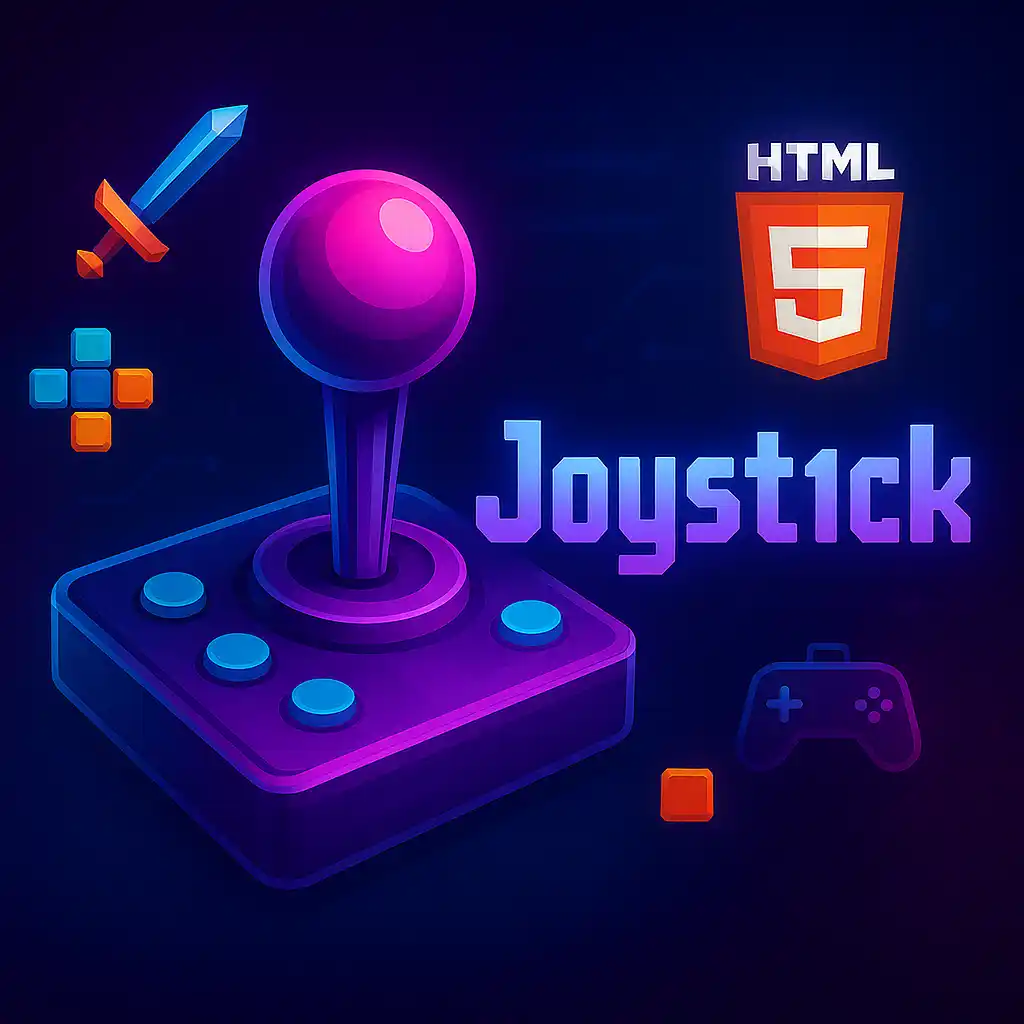