Table of Contents
Checking for Parallel Vectors in a Game’s Physics Engine
Understanding Vector Parallelism
Two vectors are considered parallel if they are scalar multiples of each other. This is a fundamental concept for optimizing physics calculations where detecting parallel vectors can simplify collision detection and other computations.
Methods for Checking Parallel Vectors
1. Cross Product Approach
The cross product of two vectors will result in a zero vector when the vectors are parallel. This can be calculated as:
Play free games on Playgama.com
Vector3 A = new Vector3(ax, ay, az);
Vector3 B = new Vector3(bx, by, bz);
Vector3 crossProduct = Vector3.Cross(A, B);
bool areParallel = crossProduct.magnitude < epsilon;
Here, epsilon
is a small threshold to account for floating point precision errors.
2. Dot Product Near Unity
After normalizing both vectors, compute their dot product. Parallel vectors will have a dot product of ±1:
A.Normalize();
B.Normalize();
float dotProduct = Vector3.Dot(A, B);
bool areParallel = Mathf.Abs(dotProduct) >= 1.0f - tolerance;
In this code, tolerance
compensates for precision errors, typically around 0.0001.
3. Scalar Multiples Identification
Check if one vector is a scalar multiple of the other. This can be done by examining the ratios of their components:
float ratioX = A.x / B.x;
float ratioY = A.y / B.y;
float ratioZ = A.z / B.z;
bool areParallel = Mathf.Approximately(ratioX, ratioY) && Mathf.Approximately(ratioY, ratioZ);
This method is sensitive to zero values in the vectors’ components.
Considerations in Implementations
- Always normalize vectors when using the dot product to ensure accurate results.
- Use appropriate thresholds to avoid issues with floating-point arithmetic.
- Consider performance implications when choosing methods, especially in real-time physics calculations.
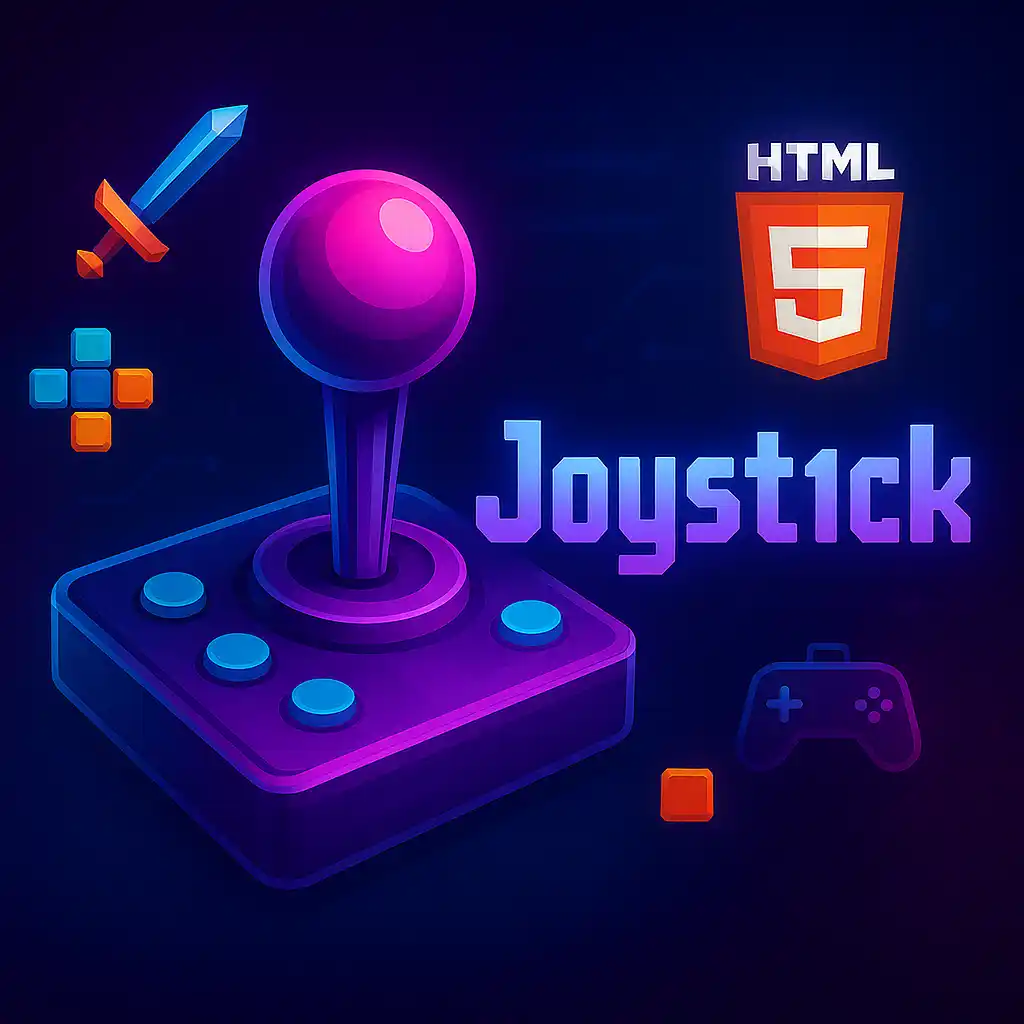